PHP Exercises: Rotate the elements of a given array of integers in left direction and return the new array
90. Rotate Array Elements Left
Write a PHP program to rotate the elements of a given array of integers (length 4 ) in left direction and return the new array.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that rotates the elements of the given array.
function test($a1)
{
// Return a new array with elements rotated by one position to the left.
return [$a1[1], $a1[2], $a1[3], $a1[0]];
}
// Create an array $a with initial values.
$a = [10, 20, -30, -40];
// Display the original array.
echo "Original array: " . implode(',', $a) . "\n";
// Call the 'test' function to rotate the array elements.
$result = test($a);
// Display the rotated array.
echo "Rotated array: " . implode(',', $result);
?>
Explanation:
- Function Definition:
- A function named test is defined with one parameter:
- $a1: an array of numbers.
- Array Rotation:
- The function returns a new array that rotates the elements of $a1 by one position to the left:
- It takes the second element $a1[1], the third element $a1[2], the fourth element $a1[3], and then the first element $a1[0], creating a new array in this order.
- Array Initialization:
- An array $a is created with the initial values: [10, 20, -30, -40].
- Display Original Array:
- The original array is displayed using echo and implode to format the array as a string:
- Function Call:
- The test function is called with the array $a, and the result is stored in the variable $result.
- Display Rotated Array:
- The rotated array is displayed using echo and implode to format the rotated array as a string:
Output:
Original array: 10,20,-30,-40 Rotated array: 20,-30,-40,10
Flowchart:
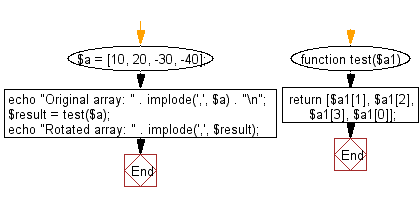
For more Practice: Solve these Related Problems:
- Write a PHP script to shift all elements in a fixed-length array one position to the left and place the first element at the end.
- Write a PHP function to perform a left rotation on an array of four integers and return the rotated array.
- Write a PHP program to simulate cyclic shifting by moving the array's head element to the tail.
- Write a PHP script to implement array rotation logic manually without using built-in rotation functions.
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to compute the sum of the elements of an given array of integers.
Next: Write a PHP program to reverse a given array of integers and length 5.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.