PHP Exercises: Reverse a given array of integers and length 5
91. Reverse Array of Length 5
Write a PHP program to reverse a given array of integers and length 5.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that reverses the order of elements in the given array.
function test($nums)
{
// Return a new array with elements in reverse order.
return [$nums[4], $nums[3], $nums[2], $nums[1], $nums[0]];
}
// Create an array $a with initial values.
$a = [10, 20, -30, -40, 50];
// Display the original array.
echo "Original array: " . implode(',', $a) . "\n";
// Call the 'test' function to reverse the array elements.
$result = test($a);
// Display the reversed array.
echo "Reverse array: " . implode(',', $result);
?>
Explanation:
- Function Definition:
- A function named test is defined with one parameter:
- $nums: an array of numbers.
- Array Reversal:
- The function returns a new array that contains the elements of $nums in reverse order:
- It explicitly selects elements in the order of index 4, 3, 2, 1, and 0, effectively reversing the array.
- Array Initialization:
- An array $a is created with the initial values: [10, 20, -30, -40, 50].
- Display Original Array:
- The original array is displayed using echo and implode to format the array as a string:
- Function Call:
- The test function is called with the array $a, and the result is stored in the variable $result.
- Display Reversed Array:
- The reversed array is displayed using echo and implode to format the reversed array as a string:
Output:
Original array: 10,20,-30,-40,50 Reverse array: 50,-40,-30,20,10
Flowchart:
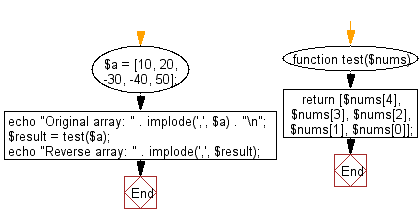
For more Practice: Solve these Related Problems:
- Write a PHP script to reverse a five-element array manually using a loop and output the reversed array.
- Write a PHP function to swap elements from both ends of a five-element array until the entire array is reversed.
- Write a PHP program to implement array reversal by iteratively exchanging elements and print the final array.
- Write a PHP script to generate the reverse order of a fixed five-element array using custom indexing.
Go to:
PREV : Rotate Array Elements Left.
NEXT : Replace All Elements with Maximum of Boundaries.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.