PHP Exercises: Find out the maximum element between the first or last element in a given array of integers, replace all elements with maximum element
92. Replace All Elements with Maximum of Boundaries
Write a PHP program to find out the maximum element between the first or last element in a given array of integers ( length 4), replace all elements with maximum element.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($nums)
{
// Find the maximum value in the array using the 'max' function
$max = max($nums);
// Return a new array containing four copies of the maximum value
return [$max, $max, $max, $max];
}
// Create an array $a with some numeric values
$a = [10, 20, -30, -40];
// Print the original array as a string using 'implode' and 'echo'
echo "Original array: " . implode(',', $a) . "\n";
// Call the 'test' function with the array $a and store the result in $result
$result = test($a);
// Print the new array with four copies of the maximum values using 'implode' and 'echo'
echo "New array with maximum values: " . implode(',', $result);
?>
Explanation:
- Function Definition:
- A function named test is defined with one parameter:
- $nums: an array of numbers.
- Finding Maximum Value:
- Inside the function, the maximum value of the array is determined using the built-in max function:
- max($nums) calculates the largest number in the array.
- Returning a New Array:
- The function returns a new array containing four copies of the maximum value:
- [$max, $max, $max, $max] creates an array with the maximum value repeated four times.
- Array Initialization:
- An array $a is created with the values: [10, 20, -30, -40].
- Display Original Array:
- The original array is displayed using echo and implode to format the array as a string:
- Function Call:
- The test function is called with the array $a, and the result is stored in the variable $result.
- Display New Array:
- The new array, which contains four copies of the maximum value, is displayed using echo and implode to format it as a string.
Output:
Original array: 10,20,-30,-40 New array with maximum values: 20,20,20,20
Flowchart:
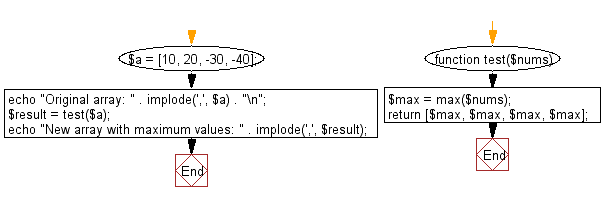
For more Practice: Solve these Related Problems:
- Write a PHP script to find the maximum between the first and last elements in an array and then replace all elements with this maximum value.
- Write a PHP function to determine the greater of an array's boundary elements and create a new array filled with that value.
- Write a PHP program to extract the first and last numbers of a four-element array and overwrite the array with their maximum.
- Write a PHP script to compare boundary values of an array and populate a new array entirely with the larger one.
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to reverse a given array of integers and length 5.
Next: Write a PHP program to create a new array containing the middle elements from the two given arrays of integers, each length 5.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.