PHP Exercises: Check a given array of integers and return true if the array contains 10 or 20 twice
97. Check for Two Occurrences of 10 or 20
Write a PHP program to check a given array of integers and return true if the array contains 10 or 20 twice. The length of the array will be 0, 1, or 2.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($nums)
{
// Return true if the array has exactly two elements and
// either both elements are 10 or both elements are 20
return sizeof($nums) == 2 && (($nums[0] == 10 && $nums[1] == 10) || ($nums[0] == 20 && $nums[1] == 20));
}
// Check and display the result of calling 'test' with the array [12, 20]
var_dump(test([12, 20]));
// Check and display the result of calling 'test' with the array [20, 20]
var_dump(test([20, 20]));
// Check and display the result of calling 'test' with the array [10, 10]
var_dump(test([10, 10]));
// Check and display the result of calling 'test' with the array [10]
var_dump(test([10]));
?>
Explanation:
- Function Definition:
- A function named test is defined, which takes one parameter:
- $nums: an array of numbers.
- Return Condition:
- The function checks two conditions:
- The array has exactly two elements (sizeof($nums) == 2).
- Either:
- Both elements are 10 ($nums[0] == 10 && $nums[1] == 10), or
- Both elements are 20 ($nums[0] == 20 && $nums[1] == 20).
- The function returns true if both conditions are met; otherwise, it returns false.
Output:
bool(false) bool(true) bool(true) bool(false)
Flowchart:
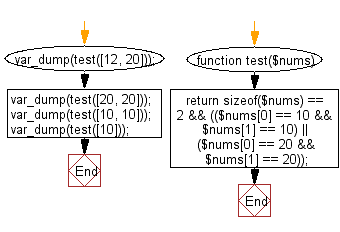
For more Practice: Solve these Related Problems:
- Write a PHP script to test if a small array of length 0 to 2 contains the number 10 or 20 exactly twice.
- Write a PHP function to iterate over an array and count occurrences of 10 and 20, returning true if either appears twice.
- Write a PHP program to check a two-element array and output true if both elements are either 10 or 20.
- Write a PHP script to use array counting functions to verify that an array contains exactly two occurrences of 10 or 20.
Go to:
PREV : Array Does Not Contain 15 or 20 Check.
NEXT : Replace 7 with 1 Following 5 in Array.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.