PHP Challenges: Find the single element appears once in an array where every element appears twice except for one
Write a PHP program to find the single element appears once in an array where every element appears twice except for one.
Input : array(5, 3, 0, 3, 0, 5, 7, 7, 9)
Sample Solution :
PHP Code :
<?php
// Function to find the single number in an array using bitwise XOR operation
function single_number($arr)
{
// Initialize the result variable
$result = 0;
// Iterate through the array
for ($i = 0; $i < sizeof($arr); $i++)
{
// Use bitwise XOR operation to find the single number
$result = $result ^ $arr[$i];
}
// Return the single number found
return $result;
}
// Define an array of numbers
$num = array(5, 3, 0, 3, 0, 5, 7, 7, 9);
// Print the single number found in the array
print_r(single_number($num) . "\n");
?>
Explanation:
Here is a brief explanation of the above PHP code:
- Function definition:
- The code defines a function named "single_number()" which finds the single number in an array using a bitwise XOR operation. It takes an array '$arr' as input.
- Inside the function:
- It initializes the variable '$result' to 0.
- It iterates through the array using a "for" loop.
- Within each iteration, it performs a bitwise XOR operation between '$result' and the current element of the array.
- After iterating through the array, the variable '$result' holds the single number as a result of XORing all the elements.
- Function Call & Testing:
- An array '$num' is defined as a set of numbers.
- The "single_number()" function is called with this array as input.
- The single number found is printed using "print_r()".
Sample Output:
9
Flowchart:
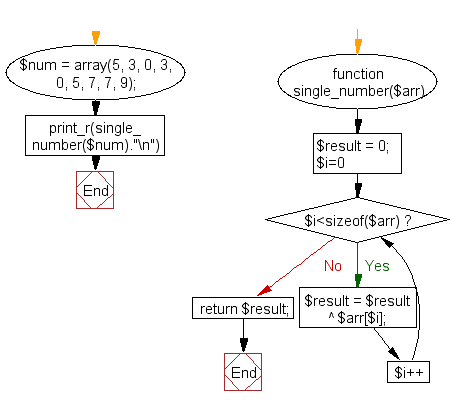
Go to:
PREV : Write a PHP program to find a single element in an array where every element appears three times except for one.
NEXT : Write a PHP program to add the digits of a positive integer repeatedly until the result has a single digit.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.