PHP Challenges: Add the digits of a positive integer repeatedly until the result has a single digit
Write a PHP program to add the digits of a positive integer repeatedly until the result has a single digit.
Input : 48
For example given number is 59, the result will be 5.
Step 1: 5 + 9 = 14
Step 2: 1 + 4 = 5
Explanation :
Sample Solution :
PHP Code :
<?php
// Function to add digits of a number until a single digit is obtained
function add_digits($num)
{
// Check if the number is positive
if ($num > 0)
{
// If positive, return the sum of digits modulo 9 plus 1
return ($num - 1) % 9 + 1;
}
else
{
// If negative or zero, return 0
return 0;
}
}
// Test the add_digits function with different inputs and print the results
print_r(add_digits(48) . "\n");
print_r(add_digits(59) . "\n");
?>
Explanation:
Here is a brief explanation of the above PHP code:
- Function definition:
- The code defines a function named "add_digits()" which calculates the digital root of a number. The digital root is obtained by repeatedly adding the digits of the number until a single-digit number is obtained.
- Inside the function:
- It checks if the input number '$num' is greater than 0.
- If the number is positive, it returns the result of the formula ($num - 1) % 9 + 1. This formula calculates the digital root by adding the digits of the number and performing modulo 9 arithmetic.
- If the number is non-positive (i.e., negative or zero), it returns 0.
- Function Call & Testing:
- The "add_digits()" function is called twice with different input numbers (48 and 59).
- he results of the function calls are printed using "print_r()", which displays the digital roots of the input numbers.
Sample Output:
3 5
Flowchart:
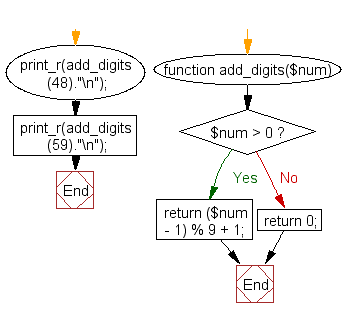
Go to:
PREV : Write a PHP program to find the single element appears once in an array where every element appears twice except for one.
NEXT : Write a PHP program to reverse the digits of an integer.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.