PHP Challenges: 3n + 1 problem
Write a PHP program where you take any positive integer n, if n is even, divide it by 2 to get n / 2. If n is odd, multiply it by 3 and add 1 to obtain 3n + 1. Repeat the process until you reach 1.
Input : 12
According to Wikipedia the Collatz conjecture is a conjecture in mathematics named after Lothar Collatz, who first proposed it in 1937. The conjecture is also known as the 3n + 1 conjecture.
The conjecture can be summarized as follows. Take any positive integer n. If n is even, divide it by 2 to get n / 2. If n is odd, multiply it by 3 and add 1 to obtain 3n + 1. Repeat the process (which has been called "Half Or Triple Plus One") indefinitely. The conjecture is that no matter what number you start with, you will always eventually reach 1.
Example :
For instance, starting with n = 12, one gets the sequence 12, 6, 3, 10, 5, 16, 8, 4, 2, 1.
n = 19, for example, takes longer to reach 1: 19, 58, 29, 88, 44, 22, 11, 34, 17, 52, 26, 13, 40, 20, 10, 5, 16, 8, 4, 2, 1.
Sample Solution :
PHP Code :
<?php
// Function to generate Collatz sequence for a given number
function collatz_sequence($x)
{
// Initialize an array to store the sequence
$num_seq = [$x];
// Check if the input is less than 1
if ($x < 1)
{
// If so, return an empty array
return [];
}
// Loop until the number becomes 1
while ($x > 1)
{
// If the number is even, divide it by 2
if ($x % 2 == 0)
{
$x = $x / 2;
}
// If the number is odd, multiply it by 3 and add 1
else
{
$x = 3 * $x + 1;
}
// Add the current number to the sequence
array_push($num_seq, $x);
}
// Return the generated sequence
return $num_seq;
}
// Test the collatz_sequence function with different inputs and print the results
print_r(collatz_sequence(12));
print_r(collatz_sequence(19));
?>
Explanation:
Here is a brief explanation of the above PHP code:
- Function definition:
- The code defines a function named "collatz_sequence()" which generates the Collatz sequence for a given number. It takes a single parameter '$x', which is the starting number of the sequence.
- Inside the function:
- It initializes an array '$num_seq' to store the sequence, starting with the given number '$x'.
- It checks if the input number is less than 1. If so, it returns an empty array as there is no Collatz sequence for numbers less than 1.
- It enters a "while"loop that continues until the number becomes 1.
- Within each iteration of the loop:
- If the number is even ($x % 2 == 0), it divides the number by 2.
- If the number is odd, it multiplies the number by 3 and adds 1.
- It adds the current number to the sequence array.
- After the loop, it returns the generated sequence.
- Function Call & Testing:
- The "collatz_sequence()" function is called twice with different input numbers (12 and 19).
- The results of the function calls are printed using print_r(), which displays the Collatz sequence generated for each input number.
Sample Output:
Array ( [0] => 12 [1] => 6 [2] => 3 [3] => 10 [4] => 5 [5] => 16 [6] => 8 [7] => 4 [8] => 2 [9] => 1 ) Array ( [0] => 19 [1] => 58 [2] => 29 [3] => 88 [4] => 44 [5] => 22 [6] => 11 [7] => 34 [8] => 17 [9] => 52 [10] => 26 [11] => 13 [12] => 40 [13] => 20 [14] => 10 [15] => 5 [16] => 16 [17] => 8 [18] => 4 [19] => 2 [20] => 1 )
Flowchart:
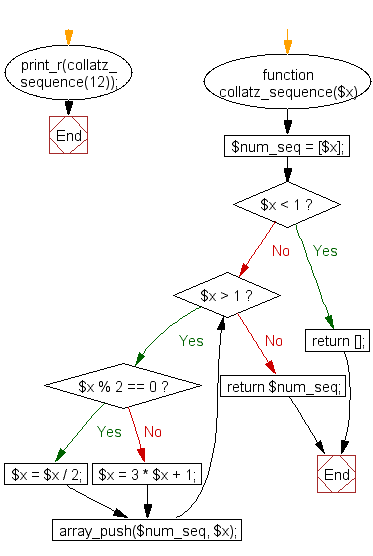
Go to:
PREV : Write a PHP program to compute the sum of the two reversed numbers and display the sum in reversed form.
NEXT : Write a PHP program to check whether a given number is an ugly number.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.