PHP Challenges: Check whether a given number is an ugly number
PHP Challenges - 1: Exercise-19 with Solution
Write a PHP program to check whether a given number is an ugly number.
Input : 12
Ugly numbers are positive numbers whose only prime factors are 2, 3 or 5. The sequence
1, 2, 3, 4, 5, 6, 8, 9, 10, 12, ...
shows the first 10 ugly numbers.
Note: 1 is typically treated as an ugly number.
Explanation :
Sample Solution :
PHP Code :
<?php
// Function to check if a number is ugly
function is_ugly($num)
{
// Store the original number in a variable
$z = $num;
// Check if the number is 0
if ($num == 0)
{
// If so, return a message indicating it's not an Ugly number
return "$num is not an Ugly number";
}
// Define an array of prime factors that make a number ugly
$x = array(2, 3, 5);
// Iterate through each prime factor
foreach ($x as $i)
{
// While the number is divisible by the current prime factor
while ($num % $i == 0)
{
// Divide the number by the prime factor
$num /= $i;
}
}
// If the number becomes 1 after dividing by all prime factors
if ($num == 1)
{
// Return a message indicating it's an Ugly number
return "$z is an Ugly number";
}
else
{
// Otherwise, return a message indicating it's not an Ugly number
return "$z is not an Ugly number";
}
}
// Test the is_ugly function with different inputs and print the results
print(is_ugly(12) . "\n");
print(is_ugly(7) . "\n");
?>
Explanation:
Here is a brief explanation of the above PHP code:
- Function definition:
- The code defines a function named "is_ugly()" which checks if a given number is an ugly number. An ugly number is a positive integer whose prime factors are only 2, 3, and 5.
- Inside the function:
- It stores the original number in a variable '$z'.
- It checks if the number is 0. If so, it returns a message indicating that it's not an ugly number.
- It defines an array '$x' containing the prime factors that make a number ugly (2, 3, and 5).
- It iterates through each prime factor in the array.
- Within each iteration, it divides the number by the current prime factor until it's no longer divisible.
- After the loop, if the number becomes 1, it returns a message indicating that it's an ugly number. Otherwise, it returns a message indicating that it's not an ugly number.
- Function Call & Testing:
- The "is_ugly()" function is called twice with different input numbers (12 and 7).
- Finally the "print()" function displays whether each input number is an ugly number or not.
Sample Output:
12 is an Ugly number 7 is not an Ugly number
Flowchart:
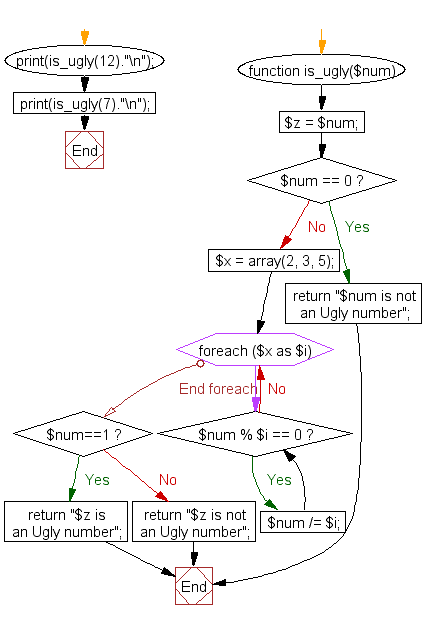
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program where you take any positive integer n, if n is even, divide it by 2 to get n / 2. If n is odd, multiply it by 3 and add 1 to obtain 3n + 1. Repeat the process until you reach 1.
Next: Write a PHP program to get the Hamming numbers upto a given numbers also check whether a given number is a Hamming number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/challenges/1/php-challenges-1-exercise-19.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics