PHP Array Exercises : Create a two-dimensional array
56. Create Two-Dimensional Array (4x4) Initialized to 10
Write a PHP script to create a two-dimensional array (4x4), initialized to 10.
Note: Use array_fill() function.
Sample Solution:
PHP Code:
<?php
// Create a multidimensional array filled with the value 10
// The outer array has 4 elements, and each element is an inner array
// The inner arrays have 4 elements each, all initialized with the value 10
$a = array_fill(0, 4, array_fill(0, 4, 10));
// Print the resulting multidimensional array
print_r($a);
?>
Output:
Array ( [0] => Array ( [0] => 10 [1] => 10 [2] => 10 [3] => 10 ) [1] => Array ( [0] => 10 [1] => 10 [2] => 10 [3] => 10 ) [2] => Array ( [0] => 10 [1] => 10 [2] => 10 [3] => 10 ) [3] => Array ( [0] => 10 [1] => 10 [2] => 10 [3] => 10 ) )
Flowchart:
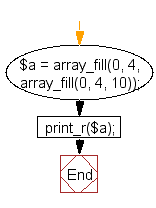
For more Practice: Solve these Related Problems:
- Write a PHP script to create a 4x4 matrix where every element is initialized to 10, and then display the matrix in a table format.
- Write a PHP function to generate a multidimensional array of arbitrary dimensions filled with a constant value.
- Write a PHP program to initialize a 4x4 array with the value 10, then modify one element and display the updated matrix.
- Write a PHP script to create a 4x4 array prefilled with a given number and then calculate the sum of all elements.
Go to:
PREV : Convert String to Array (Trim and Remove Empty Lines).
NEXT : Compare Two Multidimensional Arrays for Difference.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.