PHP Array Exercises : Combine two arrays
Write a PHP script to combine (using one array for keys and another for its values) the following two arrays.
('x', 'y', 'y'), (10, 20, 30)
Sample Solution:
PHP Code:
<?php
// Function to combine two arrays into an associative array
function combine_Array($keys, $values)
{
// Initialize an empty array to store the combined result
$result = array();
// Iterate through each element of the $keys array
foreach ($keys as $i => $k) {
// Use the keys as indices and group values accordingly
$result[$k][] = $values[$i];
}
// Use array_walk to iterate through each element in $result and modify it
array_walk($result, function (&$v) {
// If an array has only one element, replace it with that element
$v = (count($v) == 1) ? array_pop($v) : $v;
});
// Return the combined array
return $result;
}
// Two arrays to be combined
$array1 = array('x', 'y', 'y');
$array2 = array(10, 20, 30);
// Print the result of combining the arrays
print_r(combine_Array($array1, $array2));
?>
Output:
Array ( [x] => 10 [y] => Array ( [0] => 20 [1] => 30 ) )
Flowchart:
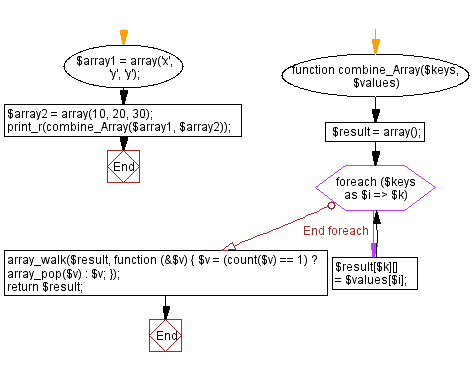
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP function to compares two multidimensional arrays and returns the difference.
Next: Write a PHP program to create a range like the specified array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.