PHP Exercises : Count number of lines in a file
16. Count Lines in File
Write a PHP script to count number of lines in a file.
Note : Store a text file name into a variable and count the number of lines of text it has.
Sample Solution:
PHP Code:
<?php
// Get the base name of the current PHP script file
$file = basename($_SERVER['PHP_SELF']);
// Count the number of lines in the current PHP script file
$no_of_lines = count(file($file));
// Display the result indicating the number of lines in the file
echo "There are $no_of_lines lines in $file"."\n";
?>
Explanation:
- Get Current File Name:
- basename($_SERVER['PHP_SELF']) retrieves the file name of the current PHP script and stores it in $file.
- Count Lines in File:
- file($file) reads all lines of the file into an array, where each array element represents one line.
- count() then counts the number of elements (lines) in this array, storing the result in $no_of_lines.
- Display Line Count:
- The echo statement outputs the number of lines in the current file with a message, like "There are 5 lines in script.php."
Output:
There are 5 lines in a6924e70-5a4c-11e7-b47b-99347412a245.php
Flowchart:
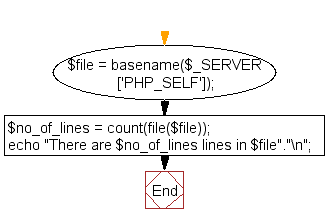
basename() function: The basename(path,suffix) function is used to get the filename from a path.
count() function: The count() function is used to count the elements of an array or the properties of an object.
Note: For objects, if you have SPL installed, you can hook into count() by implementing interface Countable. The interface has exactly one method, Countable::count(), which returns the return value for the count() function.
For more Practice: Solve these Related Problems:
- Write a PHP script to count and display the number of non-empty lines in a given text file.
- Write a PHP script to compare the line counts of two files and output the difference.
- Write a PHP script to process multiple files and generate a summary report of their line counts.
- Write a PHP script to count lines in a file and highlight any line that exceeds a specific character length.
Go to:
PREV : File Last Modified Info.
Next: Print PHP Version Without phpinfo().
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.