PHP Exercises : Swap two variables
31. Swap Variables
Write a PHP program to swap two variables.
PHP: Swapping two variables
Swapping two variables refers to mutually exchanging the values of the variables. Generally, this is done with the data in memory.
The simplest method to swap two variables is to use a third temporary variable :
define swap(a, b) temp := a a := b b := temp
Sample Solution:
Code : Swap two numbers using a third variable
PHP Code:
<?php
// Declare and initialize two variables
$a = 15;
$b = 27;
// Display the original values of the variables
echo "\nThe number before swapping is:\n";
echo "Number a =" . $a . " and b=" . $b;
// Swap Logic
$temp = $a;
$a = $b;
$b = $temp;
// Display the values after swapping
echo "\nThe number after swapping is: \n";
echo "Number a =" . $a . " and b=" . $b . "\n";
?>
Code: Swap two variables value without using third variable
<?php
// Declare and initialize two variables
$a = 15;
$b = 276;
// Display the values before swapping
echo "\nBefore swapping: ". $a . ',' . $b;
// Swap Logic using list() and array()
list($a, $b) = array($b, $a);
// Display the values after swapping
echo "\nAfter swapping: ". $a . ',' . $b."\n";
?>
Explanation:
- Variable Declaration and Initialization:
- Two variables, $a and $b, are declared and initialized with the values 15 and 27, respectively.
- Display Original Values:
- The code uses echo to output the original values of $a and $b, prefixed by the message "The number before swapping is:".
- Swap Logic:
- A temporary variable $temp is used to facilitate the swapping of values:
- The value of $a is stored in $temp.
- The value of $b is assigned to $a.
- The value stored in $temp is then assigned to $b.
- After this operation, $a now holds the value 27, and $b holds the value 15.
- Display Values After Swapping:
- The code uses echo to output the new values of $a and $b, prefixed by the message "The number after swapping is:".
Output:
The number before swapping is: Number a =15 and b=27 The number after swapping is: Number a =27 and b=15
Flowchart:
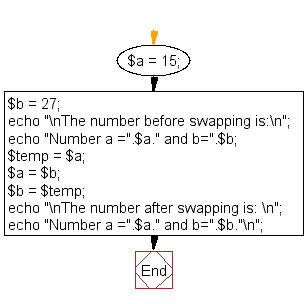
For more Practice: Solve these Related Problems:
- Write a PHP script to swap two variables without using a temporary variable by utilizing bitwise operations.
- Write a PHP script to swap variable values using array destructuring syntax and verify the swap.
- Write a PHP script to swap two variables and then test the swap using built-in assertion functions.
- Write a PHP script to perform a swap of variable values while ensuring the data types remain unchanged.
Go to:
PREV : Last Modification Time of Current Page.
NEXT : Armstrong Number Check.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.