PHP Exercises: Check whether a number is an Armstrong number or not
32. Armstrong Number Check
Write a PHP program to check whether a number is an Armstrong number or not. Return true if the number is Armstrong otherwise return false.
An Armstrong number of three digits is an integer so that the sum of the cubes of its digits is equal to the number itself. For example, 153 is an Armstrong number since 1**3 + 5**3 + 3**3 = 153
Sample Solution:
PHP Code:
<?php
// Function to check if a number is an Armstrong number
function armstrong_number($num) {
// Calculate the length of the number
$sl = strlen($num);
// Initialize a variable to store the sum of digits raised to the power of length
$sum = 0;
// Convert the number to a string for individual digit access
$num = (string)$num;
// Iterate over each digit of the number
for ($i = 0; $i < $sl; $i++) {
// Add the current digit raised to the power of length to the sum
$sum = $sum + pow((string)$num{$i}, $sl);
}
// Check if the sum is equal to the original number
if ((string)$sum == (string)$num) {
return "True";
} else {
return "False";
}
}
// Test cases
echo "Is 153 Armstrong number? " . armstrong_number(153);
echo "\nIs 21 Armstrong number? " . armstrong_number(21);
echo "\nIs 4587 Armstrong number? " . armstrong_number(4587) . "\n";
?>
Explanation:
- Function Definition:
- The function armstrong_number($num) is defined to check if a given number is an Armstrong number.
- Calculate Length of the Number:
- strlen($num) is used to calculate the length of the number (i.e., the number of digits) and store it in the variable $sl.
- Initialize Sum Variable:
- A variable $sum is initialized to 0 to store the cumulative sum of each digit raised to the power of the length of the number.
- Convert Number to String:
- The number is converted to a string using (string)$num to allow access to individual digits.
- Iterate Over Each Digit:
- A for loop iterates through each digit of the number:
- The current digit is accessed using $num{$i}.
- The digit is raised to the power of $sl using the pow() function, and this value is added to $sum.
- Check for Armstrong Condition:
- After the loop, the code checks if the calculated $sum is equal to the original number ($num).
- If they are equal, the function returns "True", indicating that it is an Armstrong number; otherwise, it returns "False".
- Test Cases:
- The function is tested with three numbers (153, 21, and 4587):
- The results are output using echo, stating whether each number is an Armstrong number or not.
Output:
Is 153 Armstrong number? True Is 21 Armstrong number? False Is 4587 Armstrong number? False
Flowchart:
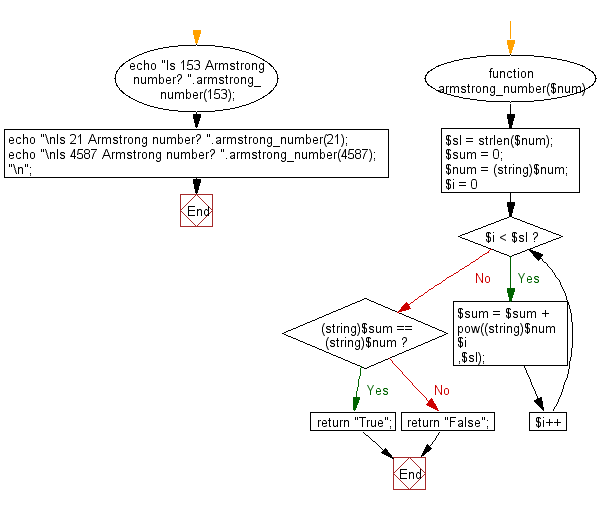
For more Practice: Solve these Related Problems:
- Write a PHP script to check for Armstrong numbers for integers of any length using dynamic loops.
- Write a PHP script to validate an array of numbers and output those that are Armstrong numbers.
- Write a PHP script to implement a function that verifies Armstrong numbers and returns a boolean result with error checking.
- Write a PHP script to print all Armstrong numbers within a specified range.
Go to:
PREV : Swap Variables.
NEXT : Convert Word to Digit.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.