PHP Exercises: Convert word to digit
33. Convert Word to Digit
Write a PHP program to convert word to digit.
Input: zero;three;five;six;eight;one
Output: 035681
Sample Solution:
PHP Code:
<?php
// Function to convert words to digits
function word_digit($word) {
// Explode the input string into an array based on the delimiter ';'
$warr = explode(';', $word);
// Initialize a variable to store the result
$result = '';
// Iterate over each word in the array
foreach ($warr as $value) {
// Switch statement to convert each word to its corresponding digit
switch (trim($value)) {
case 'zero':
$result .= '0';
break;
case 'one':
$result .= '1';
break;
case 'two':
$result .= '2';
break;
case 'three':
$result .= '3';
break;
case 'four':
$result .= '4';
break;
case 'five':
$result .= '5';
break;
case 'six':
$result .= '6';
break;
case 'seven':
$result .= '7';
break;
case 'eight':
$result .= '8';
break;
case 'nine':
$result .= '9';
break;
}
}
// Return the final result
return $result;
}
// Test cases
echo word_digit("zero;three;five;six;eight;one") . "\n";
echo word_digit("seven;zero;one") . "\n";
?>
Explanation:
- Function Definition:
- The word_digit($word) function is defined to convert a sequence of words representing numbers (e.g., "zero", "one") into their corresponding digits.
- Split Input String:
- The input string $word is split into an array $warr using explode(';', $word), with ; as the delimiter.
- Initialize Result Variable:
- A variable $result is initialized as an empty string to store the final digit sequence.
- Iterate and Convert Words to Digits:
- A foreach loop iterates through each word in $warr.
- A switch statement checks each word (after trimming whitespace) and appends the corresponding digit to $result:
- For example, if the word is "zero", 0 is appended; if "three", 3 is appended.
- Return Result:
- After the loop, the function returns $result, which now contains the converted digit sequence.
- Test Cases:
- The function is tested with two strings:
- "zero;three;five;six;eight;one" outputs 035681.
- "seven;zero;one" outputs 701.
- The results are printed using echo.
Output:
035681 701
Flowchart:
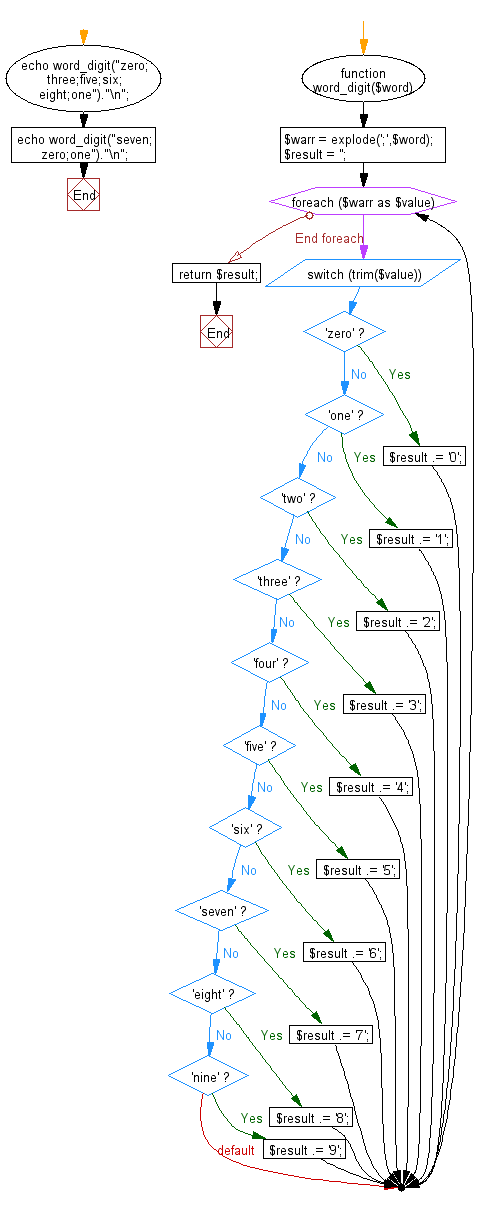
For more Practice: Solve these Related Problems:
- Write a PHP script to convert a sentence containing number words into their numeric representation.
- Write a PHP script to handle the conversion of mixed-case number words to a digit string.
- Write a PHP script to process a CSV file of number words and output the corresponding digits.
- Write a PHP script to reverse the conversion process by transforming a numeric string back into number words.
PHP Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a PHP program to check if a number is an Armstrong number or not. Return true if the number is Armstrong otherwise return false.
Next: Write a PHP program to check if the bits of the two given positions of a number are same or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.