PHP Exercises: Check if the bits of the two given positions of a number are same or not
34. Check Bits at Positions
Write a PHP program to check if the bits of the two given positions of a number are same or not.
112 - > 01110000
Test 2nd and 3rd position
Result: True
Test 4th and 5th position
Result: False
Sample Solution:
PHP Code:
<?php
// Function to test if bits at two specified positions are equal
function test_bit_position($num, $pos1, $pos2) {
// Adjust positions to start from 0 (as arrays in PHP are 0-indexed)
$pos1--;
$pos2--;
// Convert the decimal number to a binary string and reverse it
$bin_num = strrev(decbin($num));
// Check if the bits at the specified positions are equal
if ($bin_num[$pos1] == $bin_num[$pos2]) {
return "true";
} else {
return "false";
}
}
// Test case
echo test_bit_position(112, 5, 6) . "\n";
?>
Explanation:
- Function Definition:
- The test_bit_position($num, $pos1, $pos2) function checks if the bits at two specified positions in a number's binary representation are equal.
- Adjust Bit Positions:
- $pos1-- and $pos2-- adjust the positions to be zero-indexed, as PHP strings are zero-indexed (e.g., position 1 becomes 0).
- Convert Number to Binary:
- decbin($num) converts the decimal number $num to a binary string.
- strrev() reverses the binary string to match the bit positions from right to left, making it easier to access bits by index.
- Check Equality of Specified Bits:
- The function checks if the bits at the adjusted positions $pos1 and $pos2 are equal:
- If they are, it returns "true".
- If not, it returns "false".
- Test Case:
- The function is tested with the number 112 and positions 5 and 6.
- The result is printed with echo.
Output:
true
Flowchart:
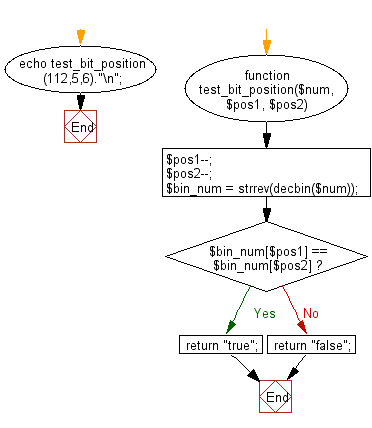
For more Practice: Solve these Related Problems:
- Write a PHP script to check if two specified bit positions in a number's binary representation are identical.
- Write a PHP script to toggle the bits at given positions and then verify their new states.
- Write a PHP script to compare bits at dynamic positions provided by user input and return a true/false result.
- Write a PHP script to create a function that checks for bit symmetry between any two positions in a binary string.
Go to:
PREV : Convert Word to Digit.
NEXT : Remove Duplicates from Sorted List.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.