PHP Exercises: Remove duplicates from a sorted list
35. Remove Duplicates from Sorted List
Write a PHP program to remove duplicates from a sorted list.
Input: (1,1,2,2,3,4,5,5)
Output: (1,2,3,4,5)
Sample Solution:
PHP Code:
<?php
// Function to remove duplicates from a list and return unique values
function remove_duplicates_list($list1) {
// Use array_unique to get unique values and array_values to re-index the array
$nums_unique = array_values(array_unique($list1));
return $nums_unique;
}
// Example usage with an array containing duplicate values
$nums = array(1, 1, 2, 2, 3, 4, 5, 5);
// Call the function and print the result
print_r(remove_duplicates_list($nums));
?>
Explanation:
- Function Definition:
- remove_duplicates_list($list1) is a function that removes duplicate values from an array and returns an array with only unique values.
- Remove Duplicates and Re-index:
- The array_unique($list1) function filters out duplicate values from the input array $list1.
- array_values() re-indexes the filtered array so that indices are sequential (starting from 0).
- The resulting unique values array is stored in $nums_unique.
- Return Unique Values:
- The function returns $nums_unique, the array with only unique values.
- Example Usage:
- An array $nums with duplicate values is defined as [1, 1, 2, 2, 3, 4, 5, 5].
- The function remove_duplicates_list($nums) is called, and the result is printed using print_r, showing only the unique values in the array.
Output:
Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 )
Flowchart:
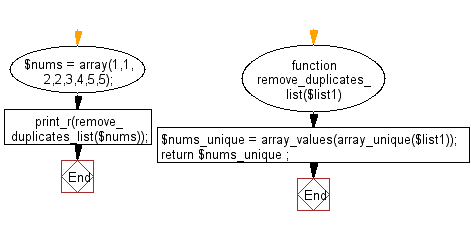
For more Practice: Solve these Related Problems:
- Write a PHP script to remove duplicates from a sorted array without using built-in array_unique() function.
- Write a PHP script to merge two pre-sorted arrays and then eliminate all duplicate values.
- Write a PHP script to remove duplicates from a sorted array and then perform a re-sort operation on the unique values.
- Write a PHP script to create a function that accepts a sorted array and returns a new array with all duplicates removed.
Go to:
PREV : Check Bits at Positions.
NEXT : Check String Suffix.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.