PHP Exercises: Find heights of the top three building in descending order from eight given buildings
PHP: Exercise-46 with Solution
Write a PHP program to find heights of the top three building in descending order from eight given buildings.
Input:
0 ≤ height of building (integer) ≤ 10,000
Pictorial Presentation:
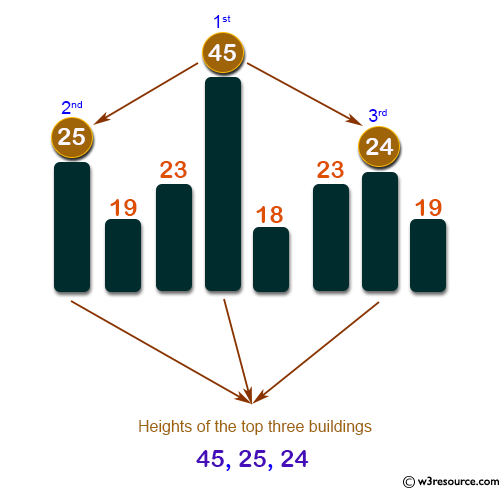
Sample Solution:
PHP Code:
<?php
// Create an empty array to store building heights
$heights = array();
// Read input from STDIN until end-of-file
while ($line = fgets(STDIN)) {
// Remove newline characters and convert to integer, then push to the heights array
rtrim($line, "\n");
array_push($heights, (int)$line);
}
// Sort the heights array in descending order
rsort($heights);
// Print the top three building heights
print("Heights of the top three buildings:\n");
echo $heights[0]."\n"; // Print the height of the tallest building
echo $heights[1]."\n"; // Print the height of the second tallest building
echo $heights[2]."\n"; // Print the height of the third tallest building
?>
Explanation:
- Array Initialization:
- An empty array called $heights is created to store the heights of buildings.
- Reading Input:
- A while loop is used to read input from the standard input (STDIN) line by line until the end-of-file (EOF) is reached.
- fgets(STDIN) reads a line of input.
- Processing Input:
- Each line is processed to remove any trailing newline characters using rtrim($line, "\n").
- The line is then converted to an integer using (int)$line and pushed into the $heights array using array_push().
- Sorting Heights:
- The rsort($heights) function sorts the $heights array in descending order, meaning the tallest buildings will appear first.
- Output Top Three Heights:
- A message is printed to indicate that the top three building heights will be displayed.
- The first three elements of the sorted $heights array (i.e., the tallest, second tallest, and third tallest buildings) are printed using echo.
- Prints:
- echo $heights[0]."\n" prints the height of the tallest building.
- echo $heights[1]."\n" prints the height of the second tallest building.
- echo $heights[2]."\n" prints the height of the third tallest building.
Output:
Heights of the top three buildings: 45 25 24
Flowchart:
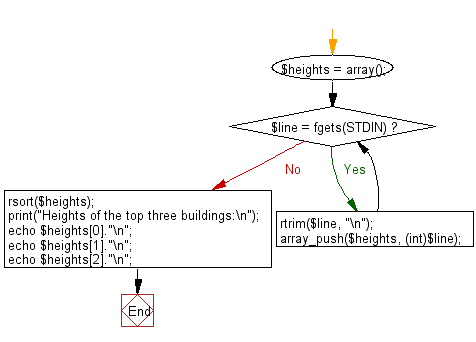
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to compute the sum of the digits of a number.
Next: Write a PHP program to compute the digit number of sum of two given integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/php-basic-exercise-46.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics