PHP Exercises: Compute the sum of the digits of a number
45. Sum of Digits
Write a PHP program to compute the sum of the digits of a number.
Sample Solution:
PHP Code:
<?php
// Define a function to calculate the sum of digits in a given number
function sum_of_digits($nums) {
// Initialize a variable to store the sum of digits
$digits_sum = 0;
// Iterate through each digit in the number
for ($i = 0; $i < strlen($nums); $i++) {
// Add the current digit to the sum
$digits_sum += $nums[$i];
}
// Return the sum of digits
return $digits_sum;
}
// Test the function with example numbers
echo sum_of_digits("12345")."\n";
echo sum_of_digits("9999")."\n";
?>
Explanation:
- Function Definition:
- The function sum_of_digits($nums) is defined to calculate the sum of the digits of a given number passed as a string.
- Initialize Sum Variable:
- A variable $digits_sum is initialized to 0 to hold the cumulative sum of the digits.
- Iterate Through Digits:
- A for loop iterates through each character in the string $nums using the length of the string (strlen($nums)) as the loop's limit.
- The loop variable $i represents the current index of the character being processed.
- Add Each Digit:
- Inside the loop, the current digit (as a character) is accessed using $nums[$i] and added to the $digits_sum variable.
- Since the digits are passed as a string, PHP automatically converts the character to its integer value when performing the addition.
- Return the Result:
- After the loop completes, the function returns the total sum of the digits.
- Test Cases:
- The function is tested with two example inputs:
- sum_of_digits("12345"), which calculates 1 + 2 + 3 + 4 + 5, resulting in 15.
- sum_of_digits("9999"), which calculates 9 + 9 + 9 + 9, resulting in 36.
- The results are printed to the output.
Output:
15 36
Flowchart:
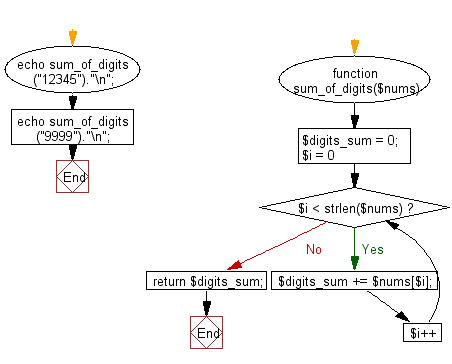
For more Practice: Solve these Related Problems:
- Write a PHP script to compute the sum of digits of a number using a recursive approach.
- Write a PHP script to calculate the sum of digits for very large numbers represented as strings.
- Write a PHP script that iterates over each digit of an integer and accumulates the total using a while loop.
- Write a PHP function to sum digits and verify correctness across multiple test cases.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to print out the sum of pairs of numbers of a given sorted array of positive integers which is equal to a given number.
Next: Write a PHP program to find heights of the top three building in descending order from eight given buildings
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.