PHP Exercises: Print out the sum of pairs of numbers of a given sorted array of positive integers that is equal to a given number
PHP: Exercise-44 with Solution
Write a PHP program to print out the sum of pairs of numbers of a given sorted array of positive integers which is equal to a given number.
Sample Solution:
PHP Code:
<?php
// Define a function to find pairs in an array that sum up to a given value
function find_Pairs($nums, $pair_sum) {
// Initialize an empty string to store pairs
$nums_pairs = "";
// Iterate through each element in the array
for ($i = 0; $i < count($nums); $i++) {
// Iterate through subsequent elements to find pairs
for ($j = $i + 1; $j < count($nums); $j++) {
// Check if the sum of the current pair equals the target sum
if ($nums[$i] + $nums[$j] == (int)$pair_sum) {
// Concatenate the pair to the result string
$nums_pairs .= $nums[$i] . "," . $nums[$j] . ";";
}
}
}
// Return the string containing pairs
return $nums_pairs;
}
// Test the function with example array and pair sums
$nums = array(0, 1, 2, 3, 4, 5, 6);
echo find_Pairs($nums, 7)."\n";
echo find_Pairs($nums, 5)."\n";
?>
Explanation:
- Function Definition:
- The function find_Pairs($nums, $pair_sum) is designed to find pairs of numbers in the array $nums that sum up to the specified value $pair_sum.
- Initialize Result Variable:
- A string variable $nums_pairs is initialized to store the pairs of numbers that meet the criteria.
- Outer Loop:
- A for loop iterates through each element in the array $nums using the index variable $i.
- Inner Loop:
- A nested for loop iterates through the subsequent elements of the array starting from the index $i + 1, using the index variable $j.
- Check for Pair Sum:
- Inside the inner loop, an if statement checks if the sum of the elements at indices $i and $j equals the target sum (int)$pair_sum.
- If they do, the pair (in the format "num1,num2;") is concatenated to the $nums_pairs string.
- Return Pairs:
- After checking all possible pairs, the function returns the string containing the pairs that add up to the target sum.
Output:
1,6;2,5;3,4; 0,5;1,4;2,3;
Flowchart:
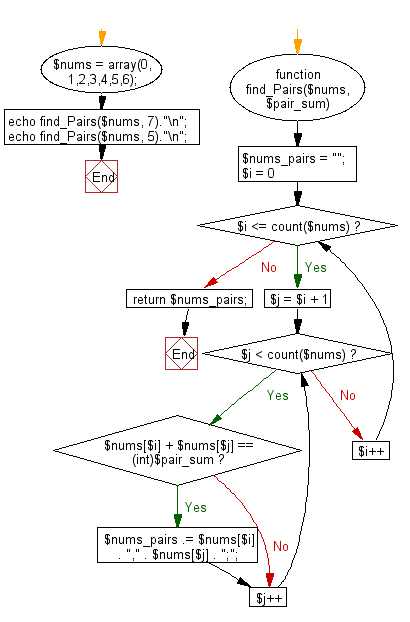
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program that multiplies corresponding elements of two given lists.
Next: Write a PHP program to compute the sum of the digits of a number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/php-basic-exercise-44.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics