PHP Exercises: Multiplies corresponding elements of two given lists
PHP: Exercise-43 with Solution
Write a PHP program that multiplies corresponding elements of two given lists.
Sample Solution:
PHP Code:
<?php
// Define a function to multiply two lists of numbers
function multiply_two_lists($x, $y)
{
// Explode the input strings into arrays using spaces as delimiters
$a = explode(' ', trim($x));
$b = explode(' ', trim($y));
// Initialize an empty array to store the multiplied values
$output = array();
// Iterate through each element of the arrays and multiply corresponding elements
foreach ($a as $key => $value) {
$output[$key] = $a[$key] * $b[$key];
}
// Return the result by imploding the array into a string
return implode(' ', $output);
}
// Test the function with example lists
echo multiply_two_lists(("10 12 3"), ("1 3 3"))."\n";
?>
Explanation:
- Define Function multiply_two_lists($x, $y):
- This function multiplies corresponding elements of two space-separated lists of numbers.
- Explode Input Strings into Arrays:
- explode(' ', trim($x)) splits the first input string $x into an array $a, using spaces as delimiters.
- explode(' ', trim($y)) does the same for the second input string $y, resulting in an array $b.
- Initialize Output Array:
- An empty array $output is created to store the results of the multiplications.
- Multiply Corresponding Elements:
- A foreach loop iterates over each element of array $a, using $key to access corresponding elements in both $a and $b.
- The multiplication is performed, and the result is stored in $output[$key].
- Return the Result as a String:
- implode(' ', $output) converts the $output array back into a space-separated string and returns it.
Output:
10 36 9
Flowchart:
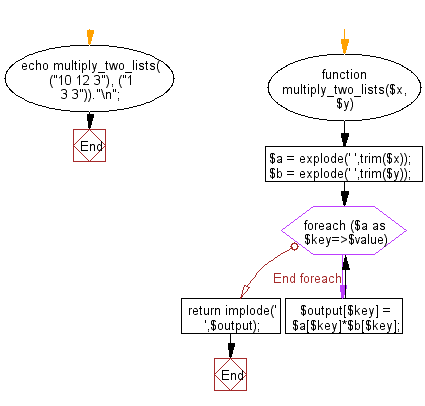
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to find the first non-repeated character in a given string.
Next: Write a PHP program to print out the sum of pairs of numbers of a given sorted array of positive integers which is equal to a given number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/php-basic-exercise-43.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics