PHP Exercises: Check whether three given lengths of three sides form a right triangle
48. Right Triangle Check
Write a PHP program to check whether three given lengths (integers) of three sides form a right triangle. Print "Yes" if the given sides form a right triangle otherwise print "No".
Input:
Integers separated by a single space.
1 ≤ length of the side ≤ 1,000
Pictorial Presentation:
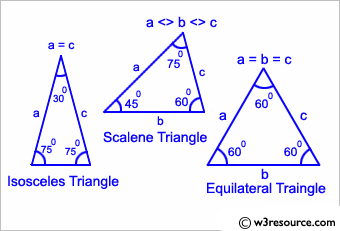
Pictorial Presentation:
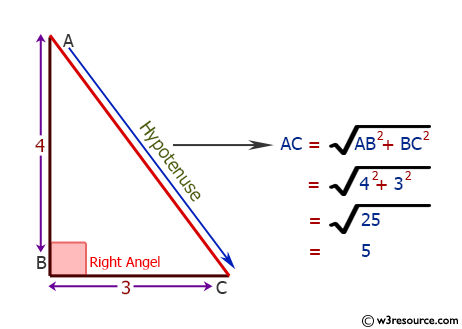
Sample Solution:
PHP Code:
<?php
// Assign values to variables representing the sides of a triangle
$a = 5;
$b = 3;
$c = 4;
// Square each side by multiplying it by itself
$a *= $a;
$b *= $b;
$c *= $c;
// Check if the sum of the squares of two sides equals the square of the third side
if ($a + $b == $c || $a + $c == $b || $b + $c == $a) {
// Print "YES" if the condition is true
echo "YES\n";
} else {
// Print "NO" if the condition is false
echo "NO\n";
}
?>
Explanation:
- Variable Assignment:
- Three variables, $a, $b, and $c, are assigned values representing the sides of a triangle: $a = 5, $b = 3, and $c = 4.
- Squaring the Sides:
- Each side is squared by multiplying it by itself:
- $a *= $a; (Now $a is 25)
- $b *= $b; (Now $b is 9)
- $c *= $c; (Now $c is 16)
- Checking the Pythagorean Theorem:
- The code checks if the sum of the squares of any two sides equals the square of the third side using the condition:
- if ($a + $b == $c || $a + $c == $b || $b + $c == $a)
- This condition evaluates whether the triangle with the given sides is a right triangle.
- Printing the Result:
- If any of the conditions are true, it prints "YES\n", indicating that the triangle can be classified as a right triangle.
- If none of the conditions are met, it prints "NO\n", indicating that the triangle is not a right triangle.
Output:
YES
Flowchart:
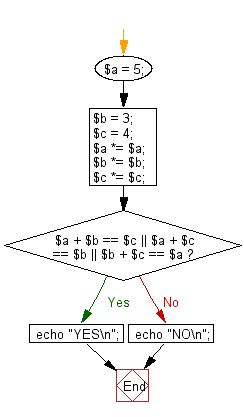
For more Practice: Solve these Related Problems:
- Write a PHP script to check if three provided side lengths form a right triangle using the Pythagorean theorem.
- Write a PHP function to verify triangle properties and test if a triangle is right-angled with floating point precision.
- Write a PHP script to compare squared values of sides to determine right angle conditions with error tolerance.
- Write a PHP script to validate triangle input and output a confirmation if it satisfies the right triangle condition.
Go to:
PREV : Digit Count of Sum.
NEXT : Solve Two-variable Equation.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.