PHP Exercises: Print the values of x, y where a, b, c, d, e and f are specified
49. Solve Two-variable Equation
Write a PHP program which solve the equation:
ax+by=c
dx+ey=f
Print the values of x, y where a, b, c, d, e and f are given.
Input:
a,b,c,d,e,f separated by a single space.
(-1,000 ≤ a,b,c,d,e,f ≤ 1,000)
Sample Solution:
PHP Code:
<?php
// Function to convert an input to a float
function to_f($e) {
return (float)$e;
}
// Read input from standard input (STDIN) line by line
while ($line = fgets(STDIN)) {
// Explode the line into an array using space as the delimiter
$a = explode(" ", $line);
// Convert each element of the array to a float using the "to_f" function
$a = array_map("to_f", $a);
// Calculate the values of x and y using the provided formulas
$x = ($a[2] * $a[4] - $a[1] * $a[5]) / ($a[0] * $a[4] - $a[3] * $a[1]);
$y = ($a[2] * $a[3] - $a[0] * $a[5]) / ($a[1] * $a[3] - $a[0] * $a[4]);
// Print the values of x and y with three decimal places
print("Values of x and y:\n");
printf("%.3f %.3f\n", $x, $y);
}
?>
Explanation:
- Function Definition:
- A function to_f($e) is defined, which converts its input to a float using (float)$e.
- Reading Input:
- A while loop reads input from standard input (STDIN) line by line using fgets(STDIN).
- Processing Input:
- Each line read is split into an array of strings using explode(" ", $line), using space as the delimiter.
- Converting Strings to Floats:
- The array_map("to_f", $a) function applies the to_f function to each element of the array $a, converting all elements to floats.
- Calculating Values of x and y:
- The values of x and y are calculated using specific mathematical formulas involving the elements of the array.
- Output:
- The results for x and y are printed with three decimal places using printf("%.3f %.3f\n", $x, $y), preceded by the message "Values of x and y:".
Output:
Values of x and y: -1.684 2.737
Flowchart:
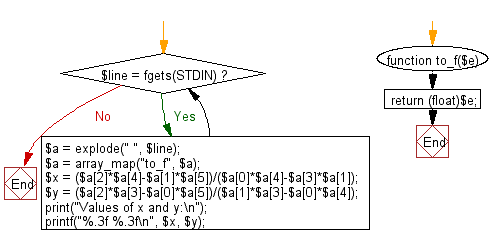
For more Practice: Solve these Related Problems:
- Write a PHP script to solve a system of two linear equations using Cramer’s Rule.
- Write a PHP function to compute x and y using determinants from the given coefficients.
- Write a PHP script to handle cases with a zero determinant and output appropriate error messages.
- Write a PHP script to validate input coefficients and compute the solution with correct rounding.
Go to:
PREV : Right Triangle Check.
NEXT : Debt Calculation in n Months.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.