PHP Exercises: Reads an integer n and find the number of combinations
51. Count Combinations of Digits
Write a PHP program which reads an integer n and find the number of combinations of a,b,c and d (0 ≤ a,b,c,d ≤ 9) where (a + b + c + d) will be equal to n.
Input:
n (1 ≤ n ≤ 50) .
Sample Solution:
PHP Code:
<?php
// Continue reading input until an empty line is encountered
while (($input = trim(fgets(STDIN))) !== '') {
// Convert the input string to an integer
$input = intval($input);
// Initialize a counter for the number of combinations
$count = 0;
// Nested loops to iterate over all possible combinations of a, b, c, and d
for ($i = 0; $i < 10; $i++) {
for ($j = 0; $j < 10; $j++) {
for ($k = 0; $k < 10; $k++) {
for ($l = 0; $l < 10; $l++) {
// Check if the sum of a, b, c, and d equals the input
if ($i + $j + $k + $l === $input) {
// Increment the counter for each valid combination
$count++;
}
}
}
}
}
// Print the number of combinations for the given input
echo "\nNumber of combinations of a, b, c, and d: ";
echo $count . "\n";
}
?>
Explanation:
- Reading Input:
- The program continuously reads input from standard input (STDIN) until an empty line is encountered using fgets(STDIN).
- Input Conversion:
- Each input string is trimmed of whitespace and converted to an integer using intval($input).
- Counter Initialization:
- A variable $count is initialized to zero to keep track of the number of valid combinations of digits.
- Nested Loops:
- Four nested for loops iterate over all possible digit combinations (from 0 to 9) for variables a, b, c, and d:
- for ($i = 0; $i < 10; $i++) iterates over possible values for a.
- for ($j = 0; $j < 10; $j++) iterates over possible values for b.
- for ($k = 0; $k < 10; $k++) iterates over possible values for c.
- for ($l = 0; $l < 10; $l++) iterates over possible values for d.
- Sum Check:
- Inside the innermost loop, the sum of the current values of i, j, k, and l is checked against the input value:
- if ($i + $j + $k + $l === $input).
- Count Increment:
- If the sum matches the input, the counter $count is incremented by one.
- Output Results:
- After all combinations have been checked, the program prints the number of valid combinations found for the given input.
Output:
Number of combinations of a,b,c and d: 56
Flowchart:
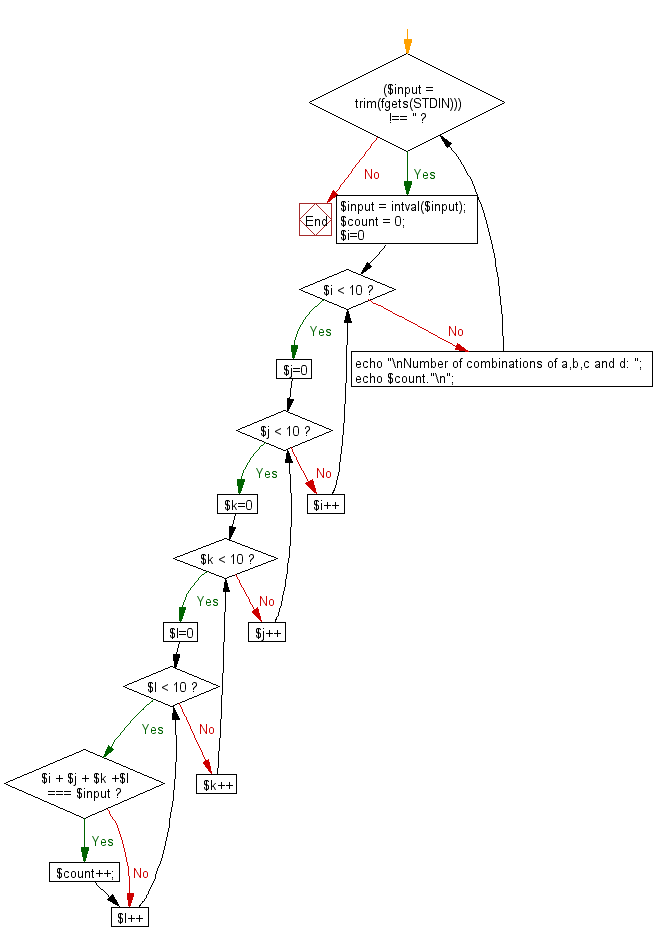
PHP Code Editor:
For more Practice: Solve these Related Problems:
- Write a PHP script to count all combinations of four digits that sum to a given integer using nested loops.
- Write a PHP function to optimize the counting of digit combinations via dynamic programming techniques.
- Write a PHP script to generate and count all unique combinations of four digits that equal a target sum.
- Write a PHP script to use recursion to count digit combinations that add up to a specified number.
Go to:
PREV : Debt Calculation in n Months.
NEXT : Count Primes Up to n.
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.