PHP Exercises: Compute and print sum of two given integers
54. Sum of Two Integers With Overflow Check
Write a PHP program to compute and print sum of two given integers (more than or equal to zero). If given integers or the sum have more than 80 digits, print "overflow".
Pictorial Presentation:
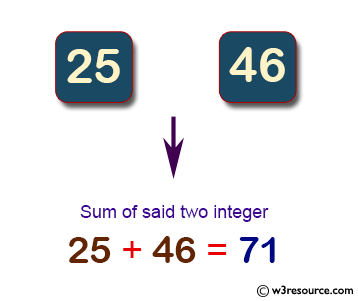
Sample Solution:
PHP Code:
<?php
// Read the number of test cases
fscanf(STDIN, '%d', $N);
// Loop through each test case
for ($i = 0; $i < $N; $i++) {
// Read two numbers as strings
fscanf(STDIN, '%s', $a);
fscanf(STDIN, '%s', $b);
// Check if the length of either number is greater than 80
if (max(strlen($a), strlen($b)) > 80) {
echo "overflow\n";
continue; // Skip to the next iteration if overflow occurs
}
// Initialize an array to store the result
$arr = array_fill(0, 81, 0);
// Pad the numbers with leading zeros to make them of length 81
$a = sprintf('%081s', $a);
$b = sprintf('%081s', $b);
// Perform addition of the two numbers
for ($j = 80; $j > 0; $j--) {
$n = $arr[$j] + $a[$j] + $b[$j];
// Check for carry
if ($n >= 10) {
$arr[$j] = substr($n, 1);
$arr[$j - 1] += 1;
} else {
$arr[$j] = $n;
}
}
// Remove leading zeros and check for overflow
$result = preg_replace('/^0+(\d+)$/', '$1', implode('', $arr));
// Output the result or indicate overflow
if (strlen($result) > 80) {
echo "overflow\n";
} else {
echo $result, PHP_EOL;
}
}
?>
Explanation:
- Read the Number of Test Cases:
- The code begins by reading an integer NNN from standard input, which indicates the number of test cases to process.
- Loop Through Each Test Case:
- A loop runs NNN times, where for each iteration:
- It reads two numbers as strings aaa and bbb.
- Check for Length Overflow:
- It checks if the length of either number is greater than 80 characters.
- If so, it outputs "overflow" and uses continue to skip to the next test case.
- Initialize Result Array:
- An array arrarrarr of size 81 is initialized to store the result of the addition, filled with zeros.
- Pad the Numbers:
- Both numbers aaa and bbb are padded with leading zeros to ensure they are exactly 81 characters long using sprintf('%081s', $a).
- Perform Addition:
- A loop iterates backward from index 80 to 1, performing the addition of corresponding digits from aaa and bbb:
- The sum nnn of the current digits and any carry from the previous addition is calculated.
- If nnn is 10 or greater, the carry is handled:
- The current position in arrarrarr is set to the last digit of nnn (using substr($n, 1)).
- The next position (to the left) is incremented by 1 to account for the carry.
- If there is no carry, the current position in arrarrarr is simply set to nnn.
- Remove Leading Zeros:
- The result is created by removing leading zeros from the final sum stored in arrarrarr using a regular expression:
- preg_replace('/^0+(\d+)$/', '$1', ...) ensures that any leading zeros are stripped.
- Output the Result:
- If the resulting number's length exceeds 80 characters after trimming, "overflow" is printed.
- Otherwise, the result is printed, followed by a newline.
Output:
46 overflow overflow overflow overflow ... overflow overflow overflow
Flowchart:
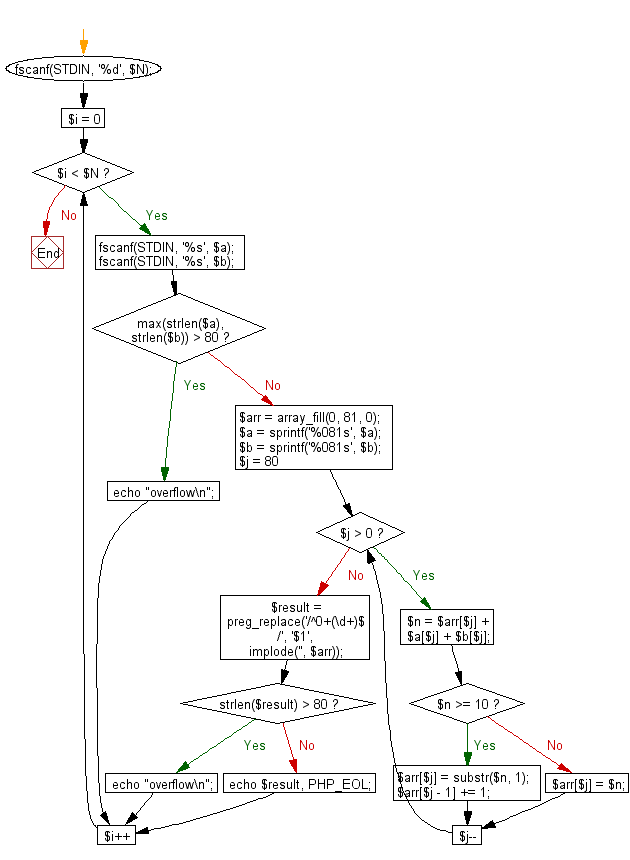
For more Practice: Solve these Related Problems:
- Write a PHP script to add two integers and output "overflow" if the result or inputs exceed 80 digits.
- Write a PHP script to perform big integer addition using strings while checking for overflow.
- Write a PHP function to validate and add two large numbers, ensuring the sum is within allowed digit limits.
- Write a PHP script to compute the sum of two integers and conditionally display an overflow message when needed.
Go to:
PREV : Circle Through 3 Points.
NEXT : Sort Six Numbers Descending.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.