PHP Exercises: Accepts six numbers as input and sorts them in descending order
55. Sort Six Numbers Descending
Write a PHP program that accepts six numbers as input and sorts them in descending order.
Input: Input consists of six numbers n1, n2, n3, n4, n5, n6 (-100000 ≤ n1, n2, n3, n4, n5, n6 ≤ 100000). The six numbers are separated by a space.
Sample Solution:
PHP Code:
<?php
// Read a line of input from STDIN and trim any leading or trailing whitespace
$stdin = trim(fgets(STDIN));
// Explode the input string into an array of integers using space as the delimiter
$nums = explode(" ", $stdin);
// Sort the array of integers in descending order
rsort($nums);
// Print a message indicating the result
print("After sorting the said integers:\n");
// Implode the sorted array into a string, separating elements with a space, and echo the result
echo implode(' ', $nums);
?>
Explanation:
- Read Input:
- The code reads a line of input from standard input (STDIN) using fgets() and trims any leading or trailing whitespace using trim().
- Explode Input into Array:
- The input string is split into an array of integers by using the explode() function with a space (" ") as the delimiter.
- Sort the Array:
- The array of integers is sorted in descending order using the rsort() function.
- Print Result Message:
- A message indicating the result of the sorting is printed: "After sorting the said integers:\n".
- Implode and Output the Sorted Array:
- The sorted array is converted back into a string using implode(), where elements are separated by a space. The resulting string is then printed to the output.
Output:
After sorting the said integers: 9 8 7 6 4 2
Flowchart:
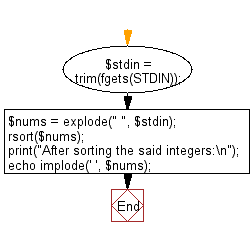
For more Practice: Solve these Related Problems:
- Write a PHP script to sort six input numbers in descending order without using built-in sort functions.
- Write a PHP script to manually implement a sorting algorithm for six numbers using nested loops.
- Write a PHP function to take six numeric inputs and output them sorted from highest to lowest using a custom sort.
- Write a PHP script to validate and then display six numbers in descending order after user input.
Go to:
PREV : Sum of Two Integers With Overflow Check.
NEXT : Test Parallel Lines.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.