PHP Exercises: Test whether two lines PQ and RS are parallel
PHP: Exercise-56 with Solution
Write a PHP program to test whether two lines PQ and RS are parallel.
The four points are P(x1, y1), Q(x2, y2), R(x3, y3), S(x4, y4).
Input: −100 ≤ x1, y1, x2, y2, x3, y3, x4, y4 ≤ 100
Each value is a real number with at most 5 digits after the decimal point.
Sample Solution:
PHP Code:
<?php
// Read the number of test cases from standard input
fscanf(STDIN, '%d', $n);
// Loop through each test case
for ($i = 0; $i < $n; $i++) {
// Read the coordinates of two points (x1, y1) and (x2, y2) for segment PQ
fscanf(STDIN, '%f %f %f %f', $x1, $y1, $x2, $y2);
// Read the coordinates of two points (x3, y3) and (x4, y4) for segment RS
fscanf(STDIN, '%f %f %f %f', $x3, $y3, $x4, $y4);
// Calculate the slopes of segments PQ and RS
$pq = INF;
if ($x2 - $x1 !== 0.0) {
$pq = ($y2 - $y1) / ($x2 - $x1);
}
$rs = INF;
if ($x4 - $x3 !== 0.0) {
$rs = ($y4 - $y3) / ($x4 - $x3);
}
// Check if the slopes are equal to determine if PQ and RS are parallel
echo $pq === $rs ? 'PQ and RS are parallel.' : 'PQ and RS are not parallel.';
echo PHP_EOL;
}
?>
Sample Input:
2
1.0 0.0 3.0 2.0 2.0 2.0 0.0 0.0
4.0 3.0 10.0 7.0 14.0 5.0 8.0 10.0
Sample Output:
PQ and RS are parallel. PQ and RS are not parallel.
Flowchart:
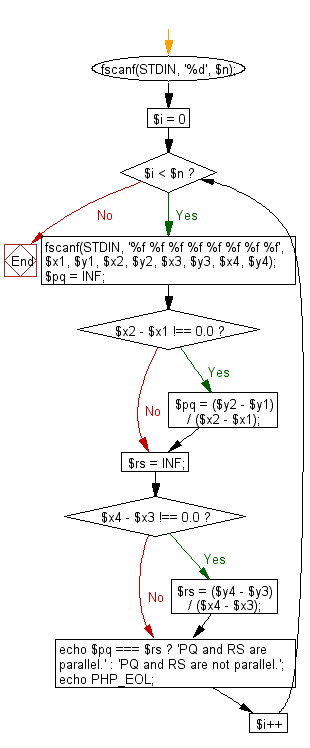
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program that accepts six numbers as input and sorts them in descending order.
Next: Write a PHP program to compute the sum of the digits of a number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics