PHP Exercises: Test if circumference of two circles intersect or overlap
PHP: Exercise-58 with Solution
There are two circles C1 with radius r1, central coordinate (x1, y1) and C2 with radius r2 and central coordinate (x2, y2)
Write a PHP program to test the followings -
"C2 is in C1" if C2 is in C1
"C1 is in C2" if C1 is in C2
"Circumference of C1 and C2 intersect" if circumference of C1 and C2 intersect, and and
"C1 and C2 do not overlap" if C1 and C2 do not overlap.
Input:Input numbers (real numbers) are separated by a space.
Input 0 to exit.
Sample Solution:
PHP Code:
<?php
// Read the number of test cases from standard input
$n = intval(fgets(STDIN));
// Loop through each test case
for ($i = 0; $i < $n; $i++) {
// Read the coordinates and radii of two circles from standard input
fscanf(STDIN, "%lf %lf %lf %lf %lf %lf", $xa, $ya, $ra, $xb, $yb, $rb);
// Calculate the distance between the centers of the two circles
$r = sqrt(($xb - $xa) * ($xb - $xa) + ($yb - $ya) * ($yb - $ya));
// Check if one circle is completely inside the other
if ($r + $ra < $rb) {
echo "C1 is in C2\n";
continue;
}
if ($r + $rb < $ra) {
echo "C2 is in C1.\n";
continue;
}
// Check if the circumferences of the circles intersect
if ($r <= $ra + $rb) {
echo "Circumference of C1 and C2 intersect.\n";
continue;
}
// Circles do not overlap or intersect
echo "C1 and C2 do not overlap.\n";
}
?>
Sample Input:
2
0.0 0.0 6.0 0.0 0.0 5.0
0.0 0.0 3.0 5.1 0.0 3.0
Sample Output:
C2 is in C1. Circumference of C1 and C2 intersect.
Flowchart:
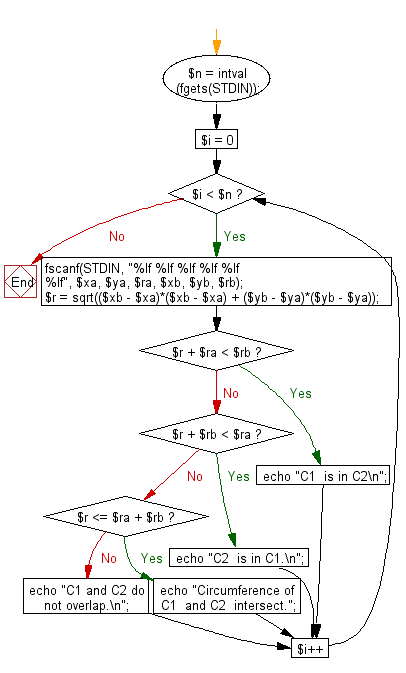
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to find the maximum sum of a contiguous subsequence from a given sequence of numbers a1, a2, a3, ... an. A subsequence of one element is also a continuous subsequence.
Next: Write a PHP program to that reads a date (from 2004/1/1 to 2004/12/31) and prints the day of the date. Jan. 1, 2004, is Friday. Note that 2004 is a leap year.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics