PHP Exercises: Find the difference between the largest integer and the smallest integer
64. Difference Between Largest and Smallest Integer Formed
Write a PHP program to find the difference between the largest integer and the smallest integer which are created by 8 numbers from 0 to 9. The number that can be rearranged shall start with 0 as in 00135668.
Input: The difference between the largest integer and the smallest integer.
Pictorial Presentation:
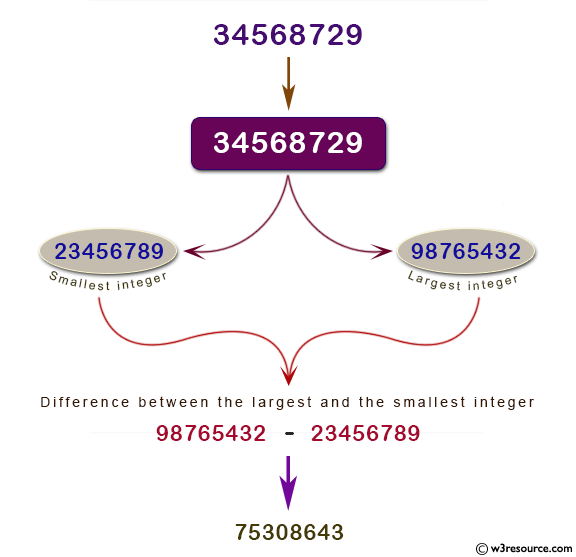
Sample Solution:
PHP Code:
<?php
// Read the number of test cases from standard input
fscanf(STDIN, '%d', $n);
// Loop through each test case
for ($i = 0; $i < $n; $i++) {
// Read the string from standard input
fscanf(STDIN, '%s', $s);
// Convert the string to an array of characters
$s = str_split($s);
// Sort the array of characters in descending order
rsort($s);
// Convert the sorted array back to an integer to get the largest number
$a = (int) implode('', $s);
// Sort the array of characters in ascending order
sort($s);
// Convert the sorted array back to an integer to get the smallest number
$b = (int) implode('', $s);
// Output the difference between the largest and smallest integers
echo "Difference between the largest integer and the smallest integer:\n";
echo $a - $b;
echo PHP_EOL;
}
?>
Explanation:
- Read Number of Test Cases:
- The code reads an integer input from standard input, representing the number of test cases (fscanf(STDIN, '%d', $n)).
- Loop Through Test Cases:
- A for loop iterates through each test case from 0 to n-1.
- Read Input String:
- For each test case, it reads a string input (fscanf(STDIN, '%s', $s)).
- Convert String to Array:
- The string is converted into an array of characters using str_split($s).
- Sort Characters in Descending Order:
- The character array is sorted in descending order using rsort($s).
- Create Largest Number:
- The sorted array is joined back into a string with implode('', $s) and then converted to an integer, representing the largest number ($a).
- Sort Characters in Ascending Order:
- The character array is sorted in ascending order using sort($s).
- Create Smallest Number:
- The sorted array is joined back into a string and converted to an integer, representing the smallest number ($b).
- Calculate and Output Difference:
- The code calculates the difference between the largest and smallest integers ($a - $b) and outputs the result, preceded by a descriptive message.
Sample Input:
1
34568729
Sample Output:
Difference between the largest integer and the smallest integer: 75308643
Flowchart:
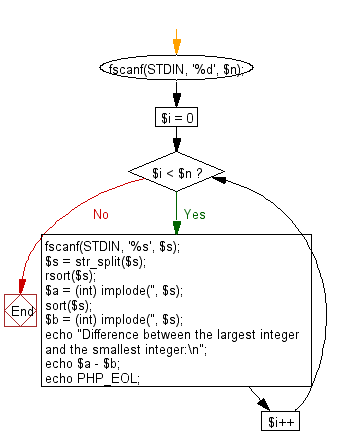
For more Practice: Solve these Related Problems:
- Write a PHP script to rearrange given digits into the largest possible integer and the smallest possible integer.
- Write a PHP function to calculate the difference between the maximum and minimum numbers formed from a set of digits.
- Write a PHP script to form extreme values from provided digits and subtract the smaller from the larger.
- Write a PHP script to rearrange digits in ascending and descending order and compute the numerical difference.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to replace a string "Python" with "PHP" and "Python" with " PHP" in a given string.
Next: Write a PHP program to compute the sum of first n given prime numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.