PHP Exercises: Find the customer number that has traded for the second consecutive for the second consecutive month from last month and the number of transactions
70. Customer Trading Analysis
Record section of ABC company records the customer number and the trading date for each month. Write a PHP program to find the customer number that has traded for the second consecutive for the second consecutive month from last month and the number of transactions.
The data of this month and the data of last month are separated by a blank line of one line and given.
Transaction format:
c1 , d1
c2 , d2
...
...
ci (1 ≤ ci ≤ 1,000) is an integer represents the customer number, di (1 ≤ di ≤ 31) is an integer represents the trading date.
Sample Solution:
PHP Code:
<?php
// Initialize an array to store the count of transactions for each customer
$x = array();
// Read input until an empty line is encountered
while (true) {
// Read a line of input and remove trailing whitespaces
$a = rtrim(fgets(STDIN));
// Break the loop if an empty line is encountered
if ($a === '') {
break;
}
// Split the input line into customer number and transaction amount
list($n, $d) = explode(',', $a);
// Initialize the transaction count for a customer if not set
if (!isset($x[$n])) {
$x[$n] = 0;
}
// Increment the transaction count for the customer
$x[$n]++;
}
// Initialize an array to store the updated transaction count for each customer
$y = array();
// Read input until an empty line is encountered
while (true) {
// Read a line of input and remove trailing whitespaces
$a = rtrim(fgets(STDIN));
// Break the loop if an empty line is encountered
if ($a === '') {
break;
}
// Split the input line into customer number and transaction amount
list($n, $d) = explode(',', $a);
// Skip if the customer number is not present in the original transactions
if (!isset($x[$n])) {
continue;
}
// Initialize the updated transaction count for a customer if not set
if (!isset($y[$n])) {
$y[$n] = $x[$n];
}
// Increment the updated transaction count for the customer
$y[$n]++;
}
// Output customer number and the number of transactions in ascending order
echo "Customer number and the number of transactions:\n";
ksort($y);
foreach ($y as $k => $v) {
echo sprintf('%d %d', $k, $v) . PHP_EOL;
}
?>
Explanation:
- Initialize Transaction Counter Array:
- $x is an array to track the number of transactions for each customer.
- Process Initial Transaction Records:
- A loop reads each line of input, removing trailing whitespaces.
- If the line is empty, it breaks the loop.
- Each line is split by a comma, with $n as the customer number and $d as the transaction amount.
- If the customer is not yet in $x, it initializes the count at 0 and then increments the transaction count for that customer.
- Initialize Updated Transaction Counter Array:
- $y is used to track transactions in a second set of transaction records.
- Process Updated Transaction Records:
- Another loop reads each line of input, following the same process as above.
- It skips customers not in the original transaction records.
- For customers in both sets, it initializes $y with the original count from $x if not already set, then increments the count.
- Output Transaction Counts in Ascending Order:
- ksort($y) sorts the customer numbers in ascending order.
- For each customer in $y, it prints the customer number and their total transaction count.
Sample Input:
125,10
55,12
34,14
125,3
55,4
125,5
Sample Output:
Customer number and the number of transactions: 55 2 125 3
Flowchart:
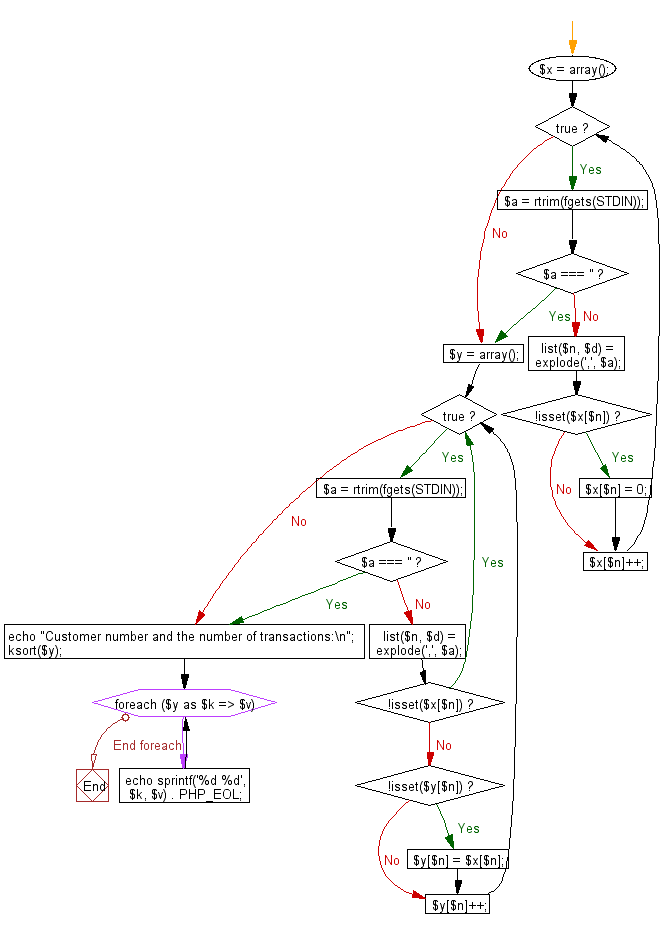
For more Practice: Solve these Related Problems:
- Write a PHP script to analyze transaction logs and identify customers with trades over consecutive months.
- Write a PHP function to process multi-line transaction data and extract customer numbers with sequential month activity.
- Write a PHP script to group and count transactions by customer, then output those with consecutive monthly trades.
- Write a PHP script to parse transaction records and report customer IDs along with the count of consecutive month trades.
Go to:
PREV : Sum of Numerical Values in Sentence.
NEXT : Island Counting.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.