PHP Exercises: Read the mass data and find the number of islands
PHP: Exercise-71 with Solution
There are 10 vertical and horizontal squares on a plane. Each square is painted blue and green. Blue represents the sea, and green represents the land. When two green squares are in contact with the top and bottom, or right and left, they are said to be ground. The area created by only one green square is called "island". For example, there are five islands in the figure below.
Write a PHP program to read the mass data and find the number of islands.
Input: A single data set is represented by 10 rows of 10 numbers representing green squares as 1 and blue squares as zeros.
Pictorial Presentation:
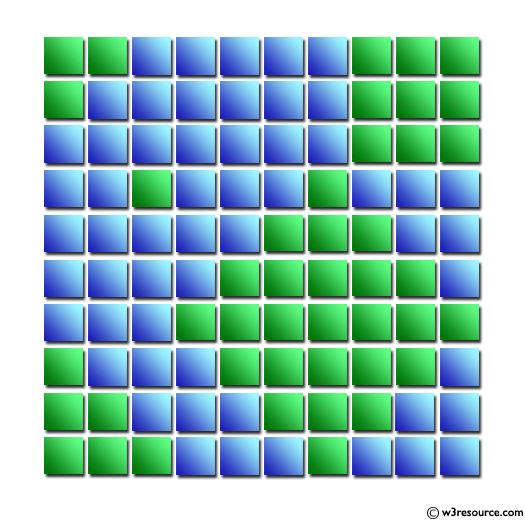
Sample Solution:
PHP Code:
<?php
// Function to perform Depth-First Search (DFS)
function dfs(&$field, $s) {
// Initialize a stack with the starting position
$stack = array($s);
// Define movement directions: right, left, down, up
$dx = array(1, -1, 0, 0);
$dy = array(0, 0, 1, -1);
// Continue DFS until the stack is empty
while (count($stack)) {
// Pop a node from the stack
$node = array_pop($stack);
// Mark the current node as visited
$field[$node[0]][$node[1]] = '0';
// Explore neighbors in all four directions
for ($i = 0; $i < 4; $i++) {
$nx = $node[1] + $dx[$i];
$ny = $node[0] + $dy[$i];
// Check if the neighbor is within the field and is unvisited
if (!isset($field[$ny][$nx]) || $field[$ny][$nx] === '0') {
continue;
}
// Push the neighbor onto the stack for further exploration
$stack[] = array($ny, $nx);
}
}
}
// Main code block
{
// Initialize a 2D array to represent the field
$field = array();
for ($i = 0; $i < 12; $i++) {
$field[] = str_split(rtrim(fgets(STDIN)));
}
// Read an extra line to handle any remaining newline characters
fscanf(STDIN, '');
// Initialize a counter for the number of islands
$c = 0;
// Iterate through the field
for ($i = 0; $i < 10; $i++) {
for ($j = 0; $j < 10; $j++) {
// If an unvisited '1' is found, perform DFS and increment the island counter
if ($field[$i][$j] === '1') {
dfs($field, array($i, $j));
$c++;
}
}
}
// Output the number of islands
echo "Number of islands: \n";
echo $c . PHP_EOL;
}
?>
Sample Input:
1100000111
1000000111
0000000111
0010001000
0000011100
0000111110
0001111111
1000111110
1100011100
1110001000
Sample Output:
Number of islands: 5
Flowchart:
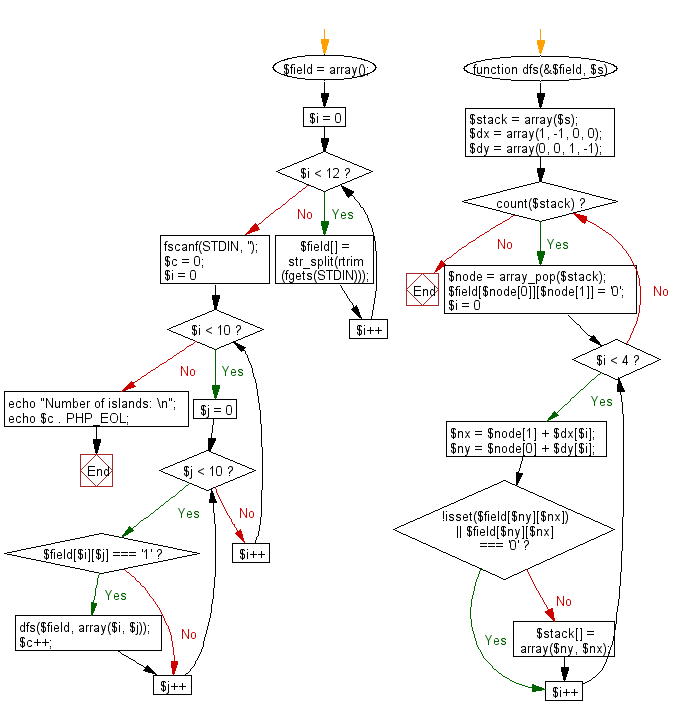
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to find the customer number that has traded for the second consecutive for the second consecutive month from last month and the number of transactions.
Next: Write a PHP program to restore the original string by entering the compressed string with this rule.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics