PHP Exercises: Cut out words of 3 to 6 characters length from a given sentence not more than 1024 characters
74. Extract Words by Length from Sentence
Internet search engine giant, such as Google accepts web pages around the world and classify them, creating a huge database. The search engines also analyze the search keywords entered by the user and create inquiries for database search. In both cases, complicated processing is carried out in order to realize efficient retrieval, but basics are all cutting out words from sentences.
Write a PHP program to cut out words of 3 to 6 characters length from a given sentence not more than 1024 characters.
Input: Multiple character strings are given. One string is given per line
Input:
English sentences consisting of delimiters and alphanumeric characters are given on one line.
Sample Solution:
PHP Code:
<?php
// Read input from standard input (stdin) and trim leading/trailing whitespaces
$stdin = trim(fgets(STDIN));
// Display the original string
echo "Original string: " . $stdin;
// Split the string into an array of words using regular expression (words are separated by spaces, commas, or periods)
$words = preg_split("/[\s,\.]/", $stdin);
// Initialize an array to store words with lengths between 3 and 6 characters
$compWords = array();
// Loop through the array of words
for ($i = 0; $i < count($words); $i++) {
// Check if the length of the current word is greater than 2 and less than 7
if (strlen($words[$i]) > 2 && strlen($words[$i]) < 7) {
// If the condition is met, add the word to the array
$compWords[] = $words[$i];
}
}
// Display the filtered words with lengths between 3 and 6 characters
echo "\nWords of 3 to 6 characters length: ";
// Print the filtered words separated by a space and add a newline
print implode(' ', $compWords) . PHP_EOL;
?>
Explanation:
- Read Input:
- Reads a line of input from the standard input, trims any leading or trailing whitespace, and stores it in $stdin.
- Display Original String:
- Prints the original input string prefixed with "Original string: ".
- Split Input into Words:
- Uses preg_split() with a regular expression to split $stdin into words, where words are separated by spaces, commas, or periods.
- Stores the resulting array of words in $words.
- Filter Words by Length:
- Initializes an empty array $compWords to hold words with lengths between 3 and 6 characters.
- Loops through $words, checking each word’s length.
- If a word’s length is greater than 2 and less than 7, it is added to $compWords.
- Display Filtered Words:
- Prints "Words of 3 to 6 characters length: " followed by the words in $compWords, separated by spaces.
- Adds a newline at the end for formatting.
Sample Input:
The quick brown fox
Sample Output:
Original string: The quick brown fox Words of 3 to 6 characters length: The quick brown fox
Flowchart:
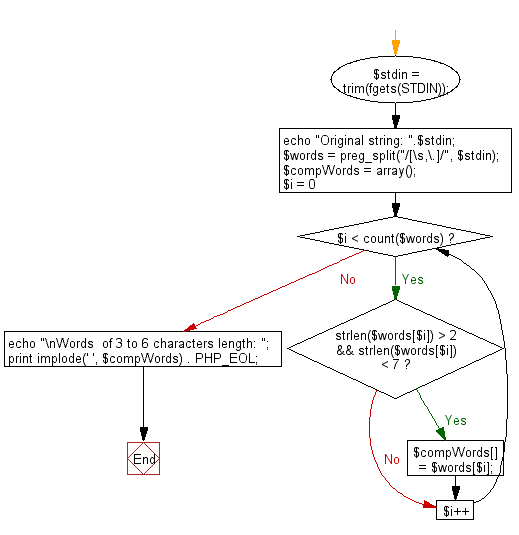
For more Practice: Solve these Related Problems:
- Write a PHP script to extract all words of length between 3 and 6 characters from a sentence using regular expressions.
- Write a PHP script to filter words by their length from a given paragraph and return only those within a defined range.
- Write a PHP script to count and list words of specified lengths from a multi-line string.
- Write a PHP script to remove words outside a 3-to-6 character length range from a sentence and reconstruct the filtered sentence.
Go to:
PREV : Convex Polygon Area Calculation.
NEXT : Maximum Path Sum in a Diamond Grid.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.