PHP Exercises: Compute the area of the polygon
73. Convex Polygon Area Calculation
A convex polygon is a simple polygon in which no line segment between two points on the boundary ever goes outside the polygon. Equivalently, it is a simple polygon whose interior is a convex set. In a convex polygon, all interior angles are less than or equal to 180 degrees, while in a strictly convex polygon all interior angles are strictly less than 180 degrees.
Write a PHP program that compute the area of the polygon . The vertices have the names vertex 1, vertex 2, vertex 3, ... vertex n according to the order of edge connections.
However, n is 3 or more and 20 or less. You can also use the following formula to calculate the area S from the lengths a, b, and c of the triangle's three sides.
Input: Multiple character strings are given. One string is given per line

Pictorial Presentation:
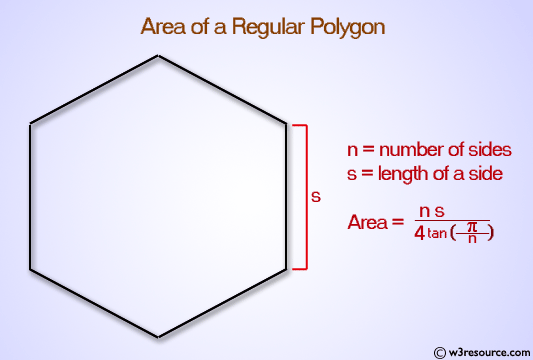
Input:
Input is given in the following format.
x1 , y1
x2 , y2
:
xn , yn
xi , yi are real numbers representing the x and y coordinates of vertex i , respectively.
Sample Solution:
PHP Code:
<?php
// Call the calc function to initiate the program
calc();
// Function to calculate the area of a polygon using the Shoelace formula
function calc() {
// Initialize an array to store Point objects
$points = array();
// Read input from standard input (stdin) and create Point objects
while ($line = trim(fgets(STDIN))) {
list($x, $y) = explode(',', $line);
$points[] = new Point($x, $y);
}
// Initialize variables for the sum and the origin point
$sum = 0;
$o = $points[0];
// Loop through the points to calculate the area of the polygon
for ($i = 1; $i < count($points) - 1; $i++) {
$p1 = $points[$i];
$p2 = $points[$i+1];
// Create a Triangle object using the origin and two consecutive points
$T = new Triangle($o, $p1, $p2);
// Add the area of the triangle to the total sum
$sum += $T->getArea();
}
// Display the calculated area of the polygon
echo "Area of the polygon:\n";
echo $sum . "\n";
}
// Class representing a Triangle with three Point objects
class Triangle {
public $A, $B, $C;
public $a, $b, $c;
// Constructor to initialize the Triangle with three Point objects
public function __construct(Point $A, Point $B, Point $C) {
$this->A = $A;
$this->B = $B;
$this->C = $C;
// Calculate the lengths of the sides of the triangle
$this->a = $B->distanceFrom($C);
$this->b = $C->distanceFrom($A);
$this->c = $A->distanceFrom($B);
}
// Method to calculate the area of the triangle using Heron's formula
public function getArea() {
$z = ($this->a + $this->b + $this->c) / 2;
return sqrt($z * ($z - $this->a) * ($z - $this->b) * ($z - $this->c));
}
}
// Class representing a Point with x and y coordinates
class Point {
public $x, $y;
// Constructor to initialize the Point with x and y coordinates
public function __construct($x, $y) {
$this->x = $x;
$this->y = $y;
}
// Method to calculate the distance between two Point objects
public function distanceFrom(Point $p) {
$dx = $this->x - $p->x;
$dy = $this->y - $p->y;
return sqrt($dx * $dx + $dy * $dy);
}
}
?>
Explanation:
- Initialize Calculation:
- The calc() function is called to start the program.
- Define calc() Function:
- Creates an empty $points array to store Point objects representing the polygon vertices.
- Reads each line from input, splits it into x and y coordinates, and adds a Point object to $points.
- Polygon Area Calculation:
- Initializes $sum to store the total area of the polygon.
- Uses the Shoelace formula by treating the polygon as a series of triangles from a fixed origin ($o = $points[0]).
- Loops through pairs of points to form triangles between the origin point ($o) and each pair of consecutive vertices ($p1, $p2).
- Creates a Triangle object for each triangle and adds its area (computed with getArea()) to $sum.
- Output the Result:
- Outputs the calculated area of the polygon.
- Triangle Class:
- Represents a triangle with points A, B, and C.
- Calculates the side lengths ($a, $b, $c) using the distanceFrom method from Point class.
- Computes the triangle’s area using Heron’s formula in the getArea() method.
- Point Class:
- Stores x and y coordinates for a point in the 2D plane.
- distanceFrom() method calculates the Euclidean distance between two points.
Sample Input:
1.0, 0.0
0.0, 0.0
1.0, 1.0
2.0, 0.0
-1.0, 1.0
Sample Output:
Area of the polygon: 1.5
Flowchart:
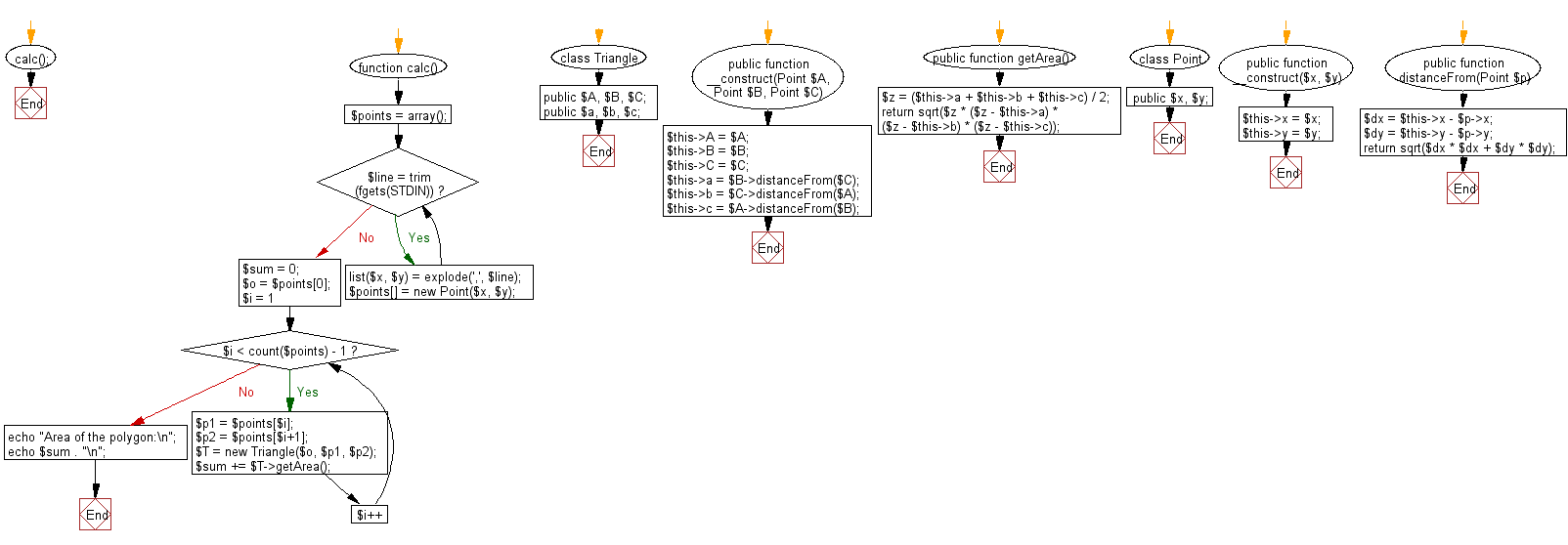
For more Practice: Solve these Related Problems:
- Write a PHP script to calculate the area of a convex polygon using the shoelace formula given its vertices.
- Write a PHP script to compute the area of a polygon by decomposing it into triangles.
- Write a PHP script to verify the convexity of a polygon before calculating its area.
- Write a PHP script to compute the area of a polygon and check if a specific point lies within it.
Go to:
PREV : Compressed String Restoration.
NEXT :
Extract Words by Length from Sentence.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.