PHP Exercises: Get the index of the last element for which the given function returns a truth value
PHP: Exercise-82 with Solution
Write a PHP program to get the index of the last element for which the given function returns a truth value.
Sample Solution:
PHP Code:
<?php
// Function definition for 'find_last_Index' that takes an array of items and a filtering function as parameters
function find_last_Index($items, $func)
{
// Use 'array_filter' to filter the items based on the given function and retrieve the keys of the filtered items
$keys = array_keys(array_filter($items, $func));
// Use 'array_pop' to retrieve and return the last key from the array of filtered keys
return array_pop($keys);
}
// Call 'find_last_Index' with an array and an anonymous function checking for odd numbers, then display the result using 'echo'
echo find_last_Index([1, 2, 3, 4], function ($n) {
return ($n % 2) === 1;
});
// Display a newline
echo "\n";
// Call 'find_last_Index' with an array and an anonymous function checking for even numbers, then display the result using 'echo'
echo find_last_Index([1, 2, 3, 4], function ($n) {
return ($n % 2) === 0;
});
?>
Explanation:
- Define find_last_Index Function:
- The function find_last_Index accepts:
- $items: an array of items to search through.
- $func: a filtering function used to determine which items to include.
- Filter Array and Retrieve Keys:
- array_filter($items, $func): Filters $items based on the condition defined in $func.
- array_keys(...): Retrieves the keys (indices) of the filtered items.
- Return the Last Key:
- array_pop($keys): Removes and returns the last key from the filtered keys array, which corresponds to the last item in $items that met the condition.
- Function Calls and Output:
- find_last_Index([1, 2, 3, 4], function ($n) { return ($n % 2) === 1; }):
- Filters for odd numbers, returns 2, the index of the last odd number (3).
- find_last_Index([1, 2, 3, 4], function ($n) { return ($n % 2) === 0; }):
- Filters for even numbers, returns 3, the index of the last even number (4).
- Purpose:
- The function find_last_Index provides a way to find and return the index of the last element in an array that meets a specified condition, enabling flexible data retrieval based on custom criteria.
Output:
2 3
Flowchart:
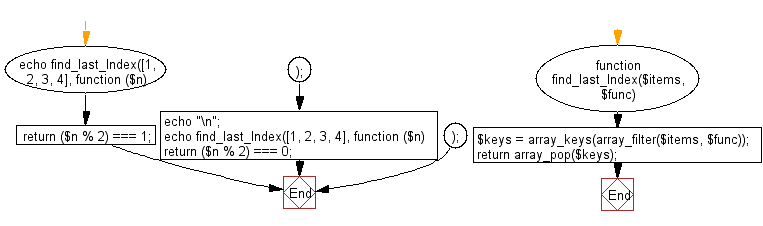
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to get the last element for which the given function returns a truth value.
Next: Write a PHP program to group the elements of an array based on the given function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/php-basic-exercise-82.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics