PHP Exercises: Get the last element for which the given function returns a truth value
PHP: Exercise-81 with Solution
Write a PHP program to get the last element for which the given function returns a truth value.
Sample Solution:
PHP Code:
<?php
// Function definition for 'find_Last' that takes an array of items and a filtering function as parameters
function find_Last($items, $func)
{
// Use 'array_filter' to filter the items based on the given function
$filteredItems = array_filter($items, $func);
// Use 'array_pop' to retrieve and return the last element from the filtered array
return array_pop($filteredItems);
}
// Call 'find_Last' with an array and an anonymous function checking for odd numbers, then display the result using 'echo'
echo find_Last([1, 2, 3, 4], function ($n) {
return ($n % 2) === 1;
});
// Display a newline
echo "\n";
// Call 'find_Last' with an array and an anonymous function checking for even numbers, then display the result using 'echo'
echo find_Last([1, 2, 3, 4], function ($n) {
return ($n % 2) === 0;
});
?>
Sample Output:
3 4
Flowchart:
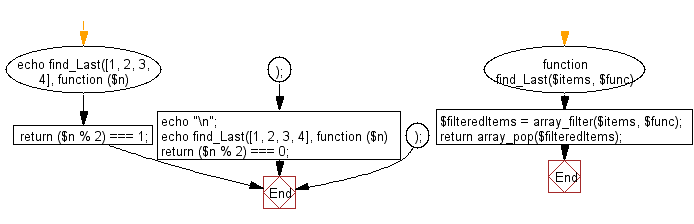
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to create a new array with n elements removed from the left.
Next: Write a PHP program to to get the index of the last element for which the given function returns a truth value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/php-basic-exercise-81.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics