PHP Exercises: Return all elements in a given array except for the first one
90. Return Array Excluding the First Element
Write a PHP program to return all elements in a given array except for the first one.
Sample Solution:
PHP Code:
<?php
// Licence: https://bit.ly/2CFA5XY
// Function definition for 'tail' that takes an array of items as a parameter
function tail($items)
{
// Use 'count' to check if the array has more than one element
// If true, use 'array_slice' to return all elements except the first one, otherwise return the original array
return count($items) > 1 ? array_slice($items, 1) : $items;
}
// Call 'tail' with an array and display the result using 'print_r'
print_r(tail([1, 2, 3]));
?>
Explanation:
- Function Definition:
- The function tail is defined to take one parameter:
- $items: an array of items.
- Counting Elements:
- The function uses count($items) to determine the number of elements in the array.
- Conditional Logic:
- If the array has more than one element (count($items) > 1):
- array_slice($items, 1) is called to return a new array containing all elements except the first one.
- If the array has one or no elements, the function returns the original array ($items).
- Purpose:
- The tail function effectively retrieves all elements of the array except the first, but if the array has only one or no elements, it returns the array as-is.
- Function Call:
- The tail function is called with the array [1, 2, 3].
- Displaying Results:
- The result of the tail function call is displayed using print_r, which shows the array excluding the first element. In this case, the output will be [2, 3].
Output:
Array ( [0] => 2 [1] => 3 )
Flowchart:
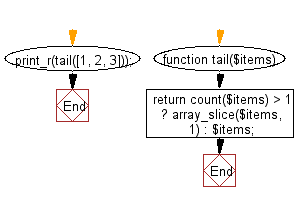
For more Practice: Solve these Related Problems:
- Write a PHP script to create a new array that excludes the first element from the original array.
- Write a PHP function to slice an array starting from the second element and output the remainder.
- Write a PHP script to extract all elements after the head of an array using array_slice.
- Write a PHP script to return a modified array with the initial element removed and display the new list.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to filter the collection using the given callback.
Next: Write a PHP program to get an array with n elements removed from the beginning of a given array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.