PHP Exercises: Get an array with n elements removed from the beginning of a given array
91. Remove n Elements from the Beginning of an Array
Write a PHP program to get an array with n elements removed from the beginning of a given array.
Sample Solution:
PHP Code:
<?php
// Licence: https://bit.ly/2CFA5XY
// Function definition for 'take' that takes an array of items and an optional parameter '$n' (default value is 1)
function take($items, $n = 1)
{
// Use 'array_slice' to return the first '$n' elements of the array
return array_slice($items, 0, $n);
}
// Call 'take' with an array and a specified number of elements to take, then display the result using 'print_r'
print_r(take([1, 2, 3], 1));
// Display a newline
echo "\n";
// Call 'take' with another array and a specified number of elements to take, then display the result using 'print_r'
print_r(take([1, 2, 3, 4, 5], 2));
?>
Explanation:
- Function Definition:
- The function take is defined to accept two parameters:
- $items: an array of items.
- $n: an optional integer (default value is 1) representing the number of elements to take from the start of the array.
- Using array_slice:
- Inside the function, array_slice($items, 0, $n) is used:
- This function returns a slice of the array, starting from index 0 and taking the first $n elements.
- Purpose:
- The take function effectively retrieves the first $n elements from the given array. If $n is not specified, it defaults to 1.
- Function Calls:
- The take function is called with different arrays and specified numbers:
- First call: take([1, 2, 3], 1) retrieves the first element, which results in [1].
- Second call: take([1, 2, 3, 4, 5], 2) retrieves the first two elements, resulting in [1, 2].
- Displaying Results:
- The results of each function call are displayed using print_r.
- A newline is printed between the two results for better readability.
Output:
Array ( [0] => 1 ) Array ( [0] => 1 [1] => 2 )
Flowchart:
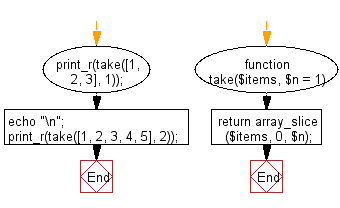
For more Practice: Solve these Related Problems:
- Write a PHP script to remove a variable number of elements from the start of an array and return the trimmed array.
- Write a PHP function to drop the first n items from an array using a custom slicing technique.
- Write a PHP script to output an array without its first n elements by iterating over the original array.
- Write a PHP script to create a new array that excludes the specified number of leftmost elements.
Go to:
PREV : Return Array Excluding the First Element.
NEXT : Filter Array to Exclude Specified Values.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.