PHP JSON Exercises : Decode a JSON string
Write a PHP script to decode a JSON string.
Sample Solution:
PHP Code:
<?php
// Define a JSON string containing key-value pairs
$json =
'{
"uglify-js": "1.3.4"
, "jshint": "0.9.1"
, "recess": "1.1.8"
, "connect": "2.1.3"
, "hogan.js": "2.0.0"
}';
// Decode the JSON string into a PHP object
var_dump(json_decode($json));
// Output a newline character for formatting
echo "\n";
// Decode the JSON string into an associative array
var_dump(json_decode($json, true));
?>
Output:
object(stdClass)#1 (5) { ["uglify-js"]=> string(5) "1.3.4" ["jshint"]=> string(5) "0.9.1" ["recess"]=> string(5) "1.1.8" ["connect"]=> string(5) "2.1.3" ["hogan.js"]=> string(5) "2.0.0" } array(5) { ["uglify-js"]=> string(5) "1.3.4" ["jshint"]=> string(5) "0.9.1" ["recess"]=> string(5) "1.1.8" ["connect"]=> string(5) "2.1.3" ["hogan.js"]=> string(5) "2.0.0" }
Explanation:
In the exercise above,
- $json = ...: Define a JSON string containing key-value pairs.
- json_decode($json): Decode the JSON string into a PHP object and return the result.
- var_dump(...): Output the result of "json_decode()" for debugging purposes.
- json_decode($json, true): Decode the JSON string into an associative array instead of an object.
- echo "\n";: Output a newline character for formatting.
Flowchart :
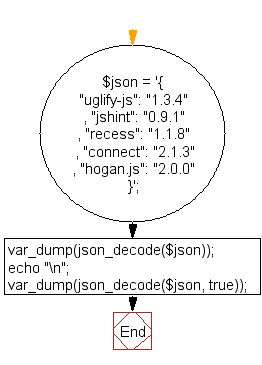
Go to:
PREV : PHP JSON Exercises Home.
NEXT :
Write a PHP script to decode large integers.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.