Python: Remove the duplicate numbers from a given list of numbers
Remove Duplicates from List
Write a Python program to remove duplicate numbers from a given list of numbers.
Sample Solution:
Python Code:
# Define a function named unique_nums that returns a list of numbers occurring only once in the given list (nums).
def unique_nums(nums):
# Use list comprehension to create a list of numbers that occur exactly once in the input list.
return [i for i in nums if nums.count(i) == 1]
# Test the function with different lists and print the results.
# Test case 1
nums = [1,2,3,2,3,4,5]
print("Original list of numbers:", nums)
print("After removing the duplicate numbers from the said list:")
print(unique_nums(nums))
# Test case 2
nums = [1,2,3,2,4,5]
print("\nOriginal list of numbers:", nums)
print("After removing the duplicate numbers from the said list:")
print(unique_nums(nums))
# Test case 3
nums = [1,2,3,4,5]
print("\nOriginal list of numbers:", nums)
print("After removing the duplicate numbers from the said list:")
print(unique_nums(nums))
Sample Output:
Original list of numbers: [1, 2, 3, 2, 3, 4, 5] After removing the duplicate numbers from the said list: [1, 4, 5] Original list of numbers: [1, 2, 3, 2, 4, 5] After removing the duplicate numbers from the said list: [1, 3, 4, 5] Original list of numbers: [1, 2, 3, 4, 5] After removing the duplicate numbers from the said list: [1, 2, 3, 4, 5]
Explanation:
Here is a breakdown of the above Python code:
- Function definition:
- The code defines a function named "unique_nums()" that returns a list of numbers occurring only once in the given list (nums).
- List comprehension:
- The function uses list comprehension to create a list of numbers (i) from the input list (nums) if the count of that number in the list is equal to 1.
- Test cases:
- The function is tested with different lists, and the results are printed using print(unique_nums(...)).
Visual Presentation:
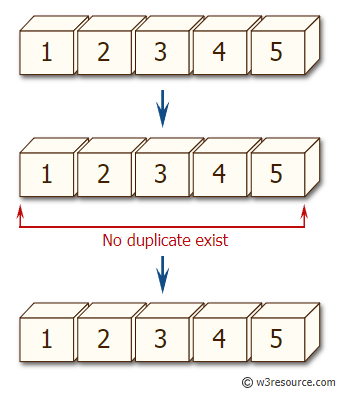
Flowchart:
For more Practice: Solve these Related Problems:
- Write a Python program to remove duplicate numbers from a list while preserving the order of elements.
- Write a Python program to filter a list of integers to include only unique values without sorting the list.
- Write a Python program to eliminate duplicates from an array using a set and list comprehension to maintain order.
- Write a Python program to create a new list containing only the unique elements from a given list.
Go to:
Previous: Write a Python program find the indices of all occurrences of a given item in a given list.
Next: Write a Python program to check whether two given circles (given center (x,y) and radius) are intersecting. Return true for intersecting otherwise false.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.