Python: Remove the first and last elements from a given string
Remove First and Last Character
Write a Python program to remove the first and last elements from a given string.
Sample Solution-1:
Python Code:
# Define a function 'test' that removes the first and last characters from a string.
def test(input_str):
# Return the original string if its length is less than 3, otherwise return the string excluding the first and last characters.
return input_str if len(input_str) < 3 else input_str[1:-1]
# Test the 'test' function with different input strings.
str1 = "PHP"
print("Original string: ", str1)
print("Removing the first and last elements from the said string: ", test(str1))
str2 = "Python"
print("\nOriginal string: ", str2)
print("Removing the first and last elements from the said string: ", test(str2))
str3 = "JavaScript"
print("\nOriginal string: ", str3)
print("Removing the first and last elements from the said string: ", test(str3))
Sample Output:
Original string: PHP Removing the first and last elements from the said string: H Original string: Python Removing the first and last elements from the said string: ytho Original string: JavaScript Removing the first and last elements from the said string: avaScrip
Explanation:
Here is a breakdown of the above Python code:
- Test Function (test function):
- The "test()" function is defined to remove the first and last characters from a given string.
- It uses a conditional expression (if-else) to check if the length of the string is less than 3.
- If the length is less than 3, the original string is returned; otherwise, a slice excluding the first and last characters is returned.
Flowchart:
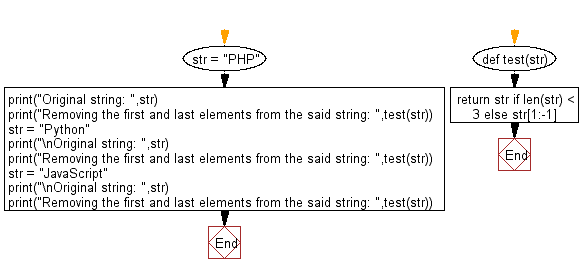
Sample Solution-2:
Python Code:
# Define a function 'test' that removes the first and last characters from a string if the length is greater than 2.
def test(input_str):
# Use slicing to remove the first and last characters if the length of the string is greater than 2; otherwise, return the original string.
return input_str[1:-1] if len(input_str) > 2 else input_str
# Test the 'test' function with different input strings.
str1 = "PHP"
print("Original string: ", str1)
print("Removing the first and last elements from the said string: ", test(str1))
str2 = "Python"
print("\nOriginal string: ", str2)
print("Removing the first and last elements from the said string: ", test(str2))
str3 = "JavaScript"
print("\nOriginal string: ", str3)
print("Removing the first and last elements from the said string: ", test(str3))
Sample Output:
Original string: PHP Removing the first and last elements from the said string: H Original string: Python Removing the first and last elements from the said string: ytho Original string: JavaScript Removing the first and last elements from the said string: avaScrip
Explanation:
Here is a breakdown of the above Python code:
- Test Function (test function):
- The "test()" function is defined to remove the first and last characters from a given string if the length is greater than 2.
- It uses a conditional expression (if-else) to check if the length of the string is greater than 2.
- If the length is greater than 2, a slice excluding the first and last characters is returned; otherwise, the original string is returned.
Flowchart:
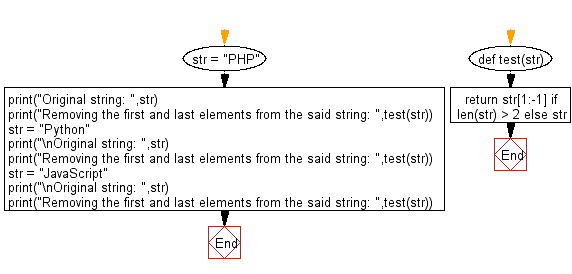
For more Practice: Solve these Related Problems:
- Write a Python program to remove the first and last characters of a string and return the remaining substring.
- Write a Python program to slice a string to exclude its first and last character.
- Write a Python program to extract the middle portion of a string by removing its first and last characters.
- Write a Python program to truncate a string by deleting the first and last elements and output the result.
Go to:
Previous: Write a Python program to check if a given string contains only lowercase or uppercase characters.
Next: Write a Python program to check if a given string contains two similar consecutive letters.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.