Python: Check whether a given month and year contains a Monday 13th
Python Basic - 1: Exercise-130 with Solution
Write a Python program to check whether a given month and year contains a Monday 13th.
Sample Solution-1:
Python Code:
# Import the 'date' class from the 'datetime' module.
from datetime import date
# Define a function 'test' that checks if the 13th day of a given month and year is a Monday.
def test(month, year):
# Create a date object for the 13th day of the given month and year.
# Use the 'strftime' method to get the day of the week (e.g., 'Monday').
is_monday_13th = date(year, month, 13).strftime("%A") == 'Monday'
# Convert the result to a string and return it.
return str(is_monday_13th)
# Provide values for the first test case (month=11, year=2022).
month = 11
year = 2022
# Print information about the first test case.
print("Month No.: ", month, " Year: ", year)
# Print the result of checking whether the 13th day of the given month and year is a Monday using the 'test' function.
print("Check whether the said month and year contain a Monday 13th.: " + test(month, year))
# Provide values for the second test case (month=6, year=2022).
month = 6
year = 2022
# Print information about the second test case.
print("\nMonth No.: ", month, " Year: ", year)
# Print the result of checking whether the 13th day of the given month and year is a Monday using the 'test' function.
print("Check whether the said month and year contain a Monday 13th.: " + test(month, year))
Sample Output:
Month No.: 11 Year: 2022 Check whether the said month and year contains a Monday 13th.: False Month No.: 6 Year: 2022 Check whether the said month and year contains a Monday 13th.: True
Explanation:
Here is a breakdown of the above Python code:
- Import Module:
- Import the 'date' class from the 'datetime' module.
- Define Test Function (test function):
- The "test()" function is defined to check if the 13th day of a given month and year is a Monday.
- It creates a date object for the 13th day of the given month and year and uses "strftime" to get the day of the week.
- The result is compared to 'Monday', and the boolean result is converted to a string.
Flowchart:
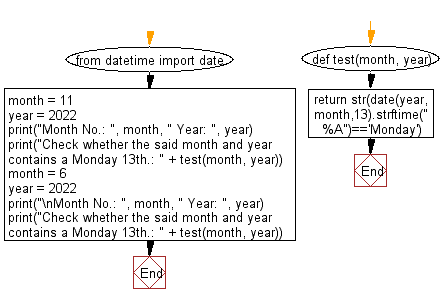
Sample Solution-2:
Python Code:
# Import the 'calendar' module to work with calendar-related functions.
import calendar
# Define a function 'test' that checks if the 13th day of a given month and year is a Monday.
def test(month, year):
# Use the 'calendar.weekday' function to get the day of the week (0 for Monday) for the 13th day of the given month and year.
is_monday_13th = calendar.weekday(year, month, 13) == 0
# Convert the result to a string and return it.
return str(is_monday_13th)
# Provide values for the first test case (month=11, year=2022).
month = 11
year = 2022
# Print information about the first test case.
print("Month No.: ", month, " Year: ", year)
# Print the result of checking whether the 13th day of the given month and year is a Monday using the 'test' function.
print("Check whether the said month and year contain a Monday 13th.: " + test(month, year))
# Provide values for the second test case (month=6, year=2022).
month = 6
year = 2022
# Print information about the second test case.
print("\nMonth No.: ", month, " Year: ", year)
# Print the result of checking whether the 13th day of the given month and year is a Monday using the 'test' function.
print("Check whether the said month and year contain a Monday 13th.: " + test(month, year))
Sample Output:
Month No.: 11 Year: 2022 Check whether the said month and year contains a Monday 13th.: False Month No.: 6 Year: 2022 Check whether the said month and year contains a Monday 13th.: True
Explanation:
Here is a breakdown of the above Python code:
- Import Module:
- Import the 'calendar' module to work with calendar-related functions.
- Define Test Function (test function):
- The "test()" function is defined to check if the 13th day of a given month and year is a Monday.
- It uses the 'calendar.weekday' function to get the day of the week (0 for Monday) for the 13th day of the given month and year.
- The result is compared to 0 (Monday), and the boolean result is converted to a string.
Flowchart:
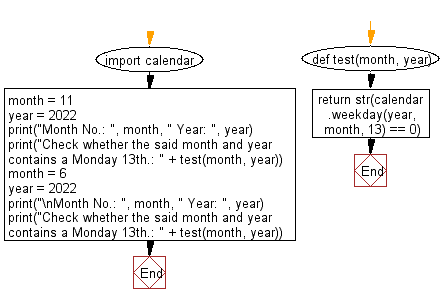
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to get the index number of all lower case letters in a given string.
Next: Write a Python program to count number of zeros and ones in the binary representation of a given integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics