Python: Compute the digit number of sum of two given integers
Python Basic - 1: Exercise-33 with Solution
Sum Digit Count
Write a Python program to compute the digit number of the sum of two given integers.
Input:
Each test case consists of two non-negative integers x and y which are separated by a space in a line.
0 ≤ x, y ≤ 1,000,000
Visual Presentation:
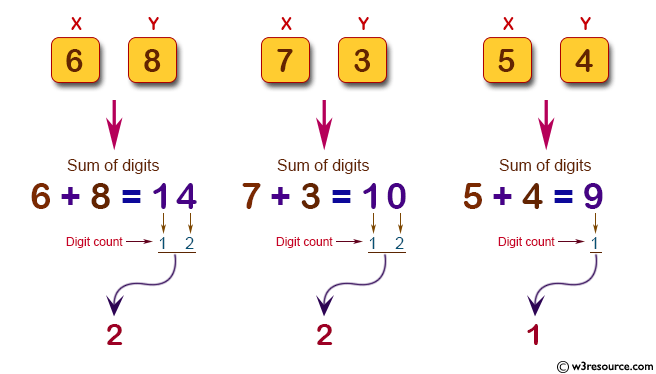
Sample Solution:
Python Code:
# Print statement to prompt the user to input two integers
print("Input two integers(a b): ")
# Using map and split to take two space-separated integers as input, then converting them to integers
a, b = map(int, input().split(" "))
# Print statement to display the number of digits in the sum of a and b
print("Number of digits of a and b:")
# Using len and str functions to find and print the length (number of digits) of the sum of a and b
print(len(str(a + b)))
Sample Output:
Input two integers(a b): 5 7 Number of digit of a and b.: 2
Explanation:
Here is the breakdown of the above Python exercise:
- Print Statement: Prompts the user to input two integers.
- Input Processing: Takes user input, expecting two space-separated integers, and converts them to integers.
- Print Statement: Indicates that it will print the number of digits in the sum of a and b.
- Calculating and Printing Digits: Converts the sum of a and b to a string, then calculates and prints the length (number of digits) of the resulting string.
Flowchart:
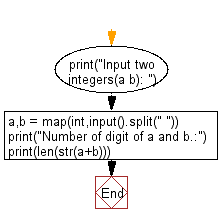
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a python program to find heights of the top three building in descending order from eight given buildings.
Next: Write a Python program to check whether three given lengths (integers) of three sides form a right triangle. Print "Yes" if the given sides form a right triangle otherwise print "No".
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/basic/python-basic-1-exercise-33.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics