Python: Accepts six numbers as input and sorts them in descending order
Python Basic - 1: Exercise-42 with Solution
Sort Six Numbers
Write a Python program that accepts six numbers as input and sorts them in descending order.
Input:
Input consists of six numbers n1, n2, n3, n4, n5, n6 (-100000 ≤ n1, n2, n3, n4, n5, n6 ≤ 100000). The six numbers are separated by a space.
Input six integers:
15 30 25 14 35 40
After sorting the said integers:
40 35 30 25 15 14
Visual Presentation:
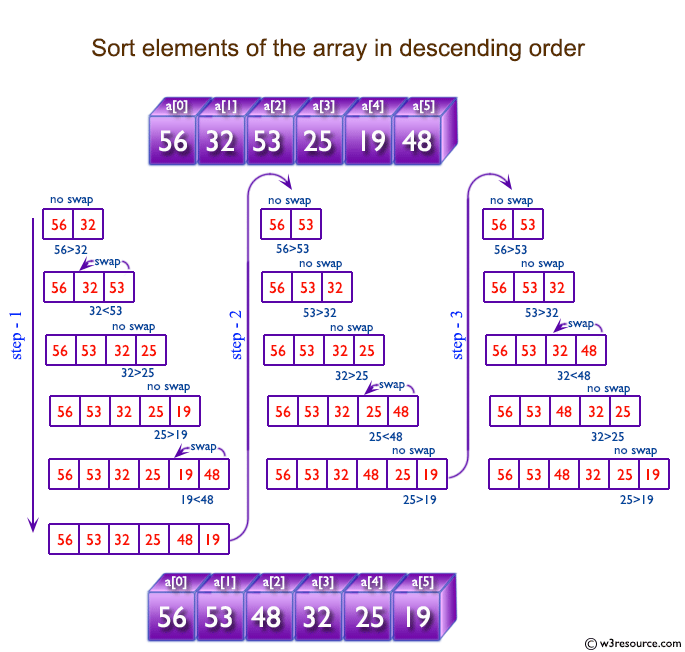
Sample Solution:
Python Code:
# Print statement to prompt the user to input six integers
print("Input six integers:")
# Take user input, split it into a list of integers, and assign it to the variable 'nums'
nums = list(map(int, input().split()))
# Sort the list of integers in ascending order
nums.sort()
# Reverse the order of the sorted list to get descending order
nums.reverse()
# Print statement to indicate the result after sorting the integers
print("After sorting the said integers:")
# Unpack and print the sorted integers
print(*nums)
Sample Output:
Input six integers: 15 30 25 14 35 40 After sorting the said ntegers: 40 35 30 25 15 14
Explanation:
Here is a breakdown of the above Python code:
- The code starts by printing a message to prompt the user to input six integers.
- It then takes user input, splits it into a list of integers, and assigns it to the variable 'nums'.
- The list of integers (nums) is sorted in ascending order using the "sort()" method.
- The order of the sorted list is reversed using the "reverse()" method, resulting in descending order.
- Another print statement is used to indicate the result after sorting the integers.
- Finally, the sorted integers are unpacked and printed using the print(*nums) statement.
Flowchart:
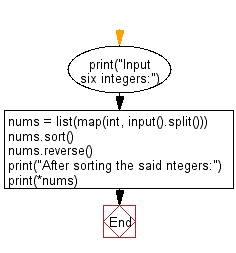
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to compute and print sum of two given integers (more than or equal to zero). If given integers or the sum have more than 80 digits, print "overflow".
Next: Write a Python program to test whether two lines PQ and RS are parallel. The four points are P(x1, y1), Q(x2, y2), R(x3, y3), S(x4, y4).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/basic/python-basic-1-exercise-42.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics