Python: Reads a text and prints two word
Word Frequency and Length
Write a Python program that reads text (only alphabetical characters and spaces) and prints two words. The first word is the one that appears most often in the text. The second one is the word with the most letters.
Note: A word is a sequence of letters which is separated by the spaces.
Input:A text is given in a line with following condition:
a. The number of letters in the text is less than or equal to 1000.
b. The number of letters in a word is less than or equal to 32.
c. There is only one word which is arise most frequently in given text.
d. There is only one word which has the maximum number of letters in given text.
Input text: Thank you for your comment and your participation.
Output: your participation.
Input:
Input text: Thank you for your comment and your participation.
Output: your participation.
Visual Presentation:
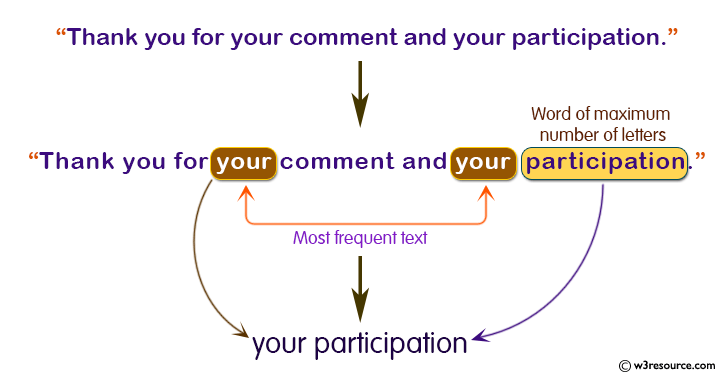
Sample Solution:
Python Code:
# Import the 'collections' module
import collections
# Prompt the user to input a text in a line
print("Input a text in a line.")
# Take user input, split it into a list of words, and convert each word to a string
text_list = list(map(str, input().split()))
# Use Counter to count the occurrences of each word in the text
sc = collections.Counter(text_list)
# Find the most common word in the text
common_word = sc.most_common()[0][0]
# Initialize an empty string to store the word with the maximum number of letters
max_char = ""
# Iterate through each word in the text
for s in text_list:
# Check if the length of the current word is greater than the length of 'max_char'
if len(max_char) < len(s):
max_char = s # Update 'max_char' with the current word
# Print the most frequent text and the word with the maximum number of letters
print("\nMost frequent text and the word which has the maximum number of letters.")
print(common_word, max_char)
Sample Output:
Input a text in a line. Thank you for your comment and your participation. Most frequent text and the word which has the maximum number of letters. your participation.
Explanation:
Here is a breakdown of the above Python code:
- First the code imports the 'collections' module.
- It prompts the user to input a text in a line.
- The input text is split into a list of words, and each word is converted to a string.
- The Counter is used to count the occurrences of each word in the text.
- The most common word in the text is obtained using sc.most_common().
- The code iterates through each word in the text to find the word with the maximum number of letters.
- The most frequent text and the word with the maximum number of letters are printed.
Flowchart:
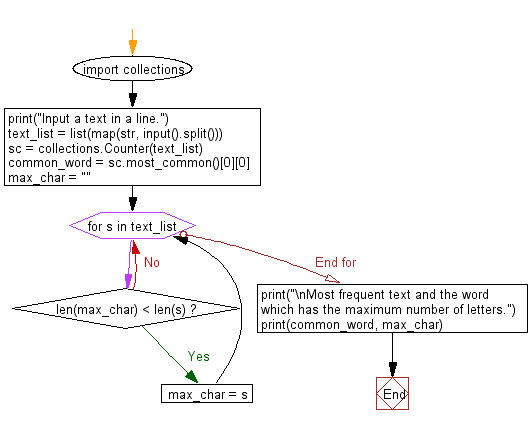
For more Practice: Solve these Related Problems:
- Write a Python program to find the most common starting letter among all words in a given text.
- Write a Python program to identify the longest word that appears more than once in the provided text.
- Write a Python program to create a frequency distribution of word lengths from a given paragraph.
- Write a Python program to find all words that occur only once in a given text.
Go to:
Previous: Write a Python program to that reads a date (from 2016/1/1 to 2016/12/31) and prints the day of the date. Jan. 1, 2016, is Friday. Note that 2016 is a leap year.
Next: Write a Python program that reads n digits (given) chosen from 0 to 9 and prints the number of combinations where the sum of the digits equals to another given number (s). Do not use the same digits in a combination.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.