Python: Reads a date and prints the day of the date
Python Basic - 1: Exercise-46 with Solution
Day of Date Finder
Write a Python program that reads a date (from 2016/1/1 to 2016/12/31) and prints the day of the date. Jan. 1, 2016, is Friday. Note that 2016 is a leap year.
Input:
Two integers m and d separated by a single space in a line, m ,d represent the month and the day.
Visual Presentation:
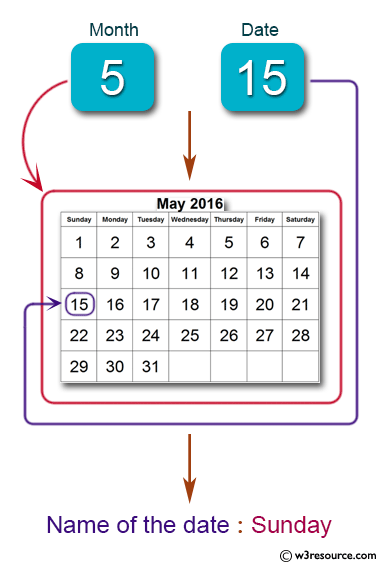
Sample Solution:
Python Code:
# Import the 'date' class from the 'datetime' module
from datetime import date
# Prompt the user to input the month and date separated by a single space
print("Input month and date (separated by a single space):")
# Take user input for month and date, and convert them to integers
m, d = map(int, input().split())
# Define a dictionary mapping weekdays' numeric representation to their names
weeks = {1: 'Monday', 2: 'Tuesday', 3: 'Wednesday', 4: 'Thursday', 5: 'Friday', 6: 'Saturday', 7: 'Sunday'}
# Calculate the ISO weekday for the given date using 'date.isoweekday' method
w = date.isoweekday(date(2016, m, d))
# Print the name of the date based on the calculated weekday
print("Name of the date: ", weeks[w])
Sample Output:
Input month and date (separated by a single space): 5 15 Name of the date: Sunday
Explanation:
Here is a breakdown of the above Python code:
- The code imports the "date" class from the "datetime" module.
- It prompts the user to input the month and date separated by a single space.
- The input for the month and date is taken, and both are converted to integers.
- A dictionary (weeks) is defined to map numeric representations of weekdays to their names.
- The ISO weekday for the given date (2016, m, d) is calculated using the date.isoweekday method.
- The code prints the name of the date based on the calculated weekday.
Flowchart:
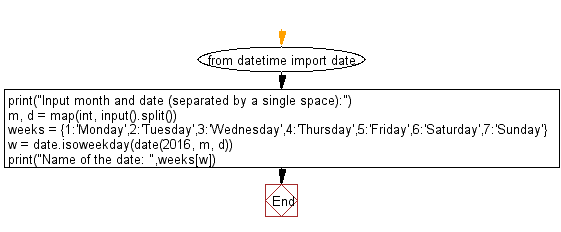
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to test if circumference of two circles intersect or overlap.
Next: Write a Python program which reads a text (only alphabetical characters and spaces.) and prints two words. The first one is the word which is arise most frequently in the text. The second one is the word which has the maximum number of letters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/basic/python-basic-1-exercise-46.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics