Python: Check whether a given string is an "isogram" or not
Isogram Checker
From Wikipedia:
An isogram (also known as a "nonpattern word") is a logological term for a word or phrase without a repeating letter. It is also used by some people to mean a word or phrase in which each letter appears the same number of times, not necessarily just once. Conveniently, the word itself is an isogram in both senses of the word, making it autological.
Write a Python program to check whether a given string is an "isogram" or not.
Sample Solution:
Python Code:
# Define a function to check if a string is an isogram
def check_isogram(str1):
# Convert the string to lowercase and check if its length is equal to the length of its set
return len(str1) == len(set(str1.lower()))
# Test cases
print(check_isogram("w3resource"))
print(check_isogram("w3r"))
print(check_isogram("Python"))
print(check_isogram("Java"))
Sample Output:
False True True False
Explanation:
Here is a breakdown of the above Python code:
- The function "check_isogram()" takes a string 'str1' as input.
- It converts the string to lowercase using "lower()" to make the comparison case-insensitive.
- It checks if the length of the string is equal to the length of its set, ensuring all characters are unique.
- The function returns 'True' if the string is an isogram and 'False' otherwise.
- Test cases demonstrate the function's functionality for different input strings.
Visual Presentation:
Flowchart:
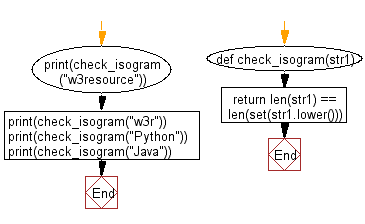
For more Practice: Solve these Related Problems:
- Write a Python program to check if a given word is an isogram by ensuring each letter appears only once.
- Write a Python program to determine if a string is an isogram irrespective of letter case.
- Write a Python program to verify that a word contains no repeating characters using a frequency dictionary.
- Write a Python program to assess whether a phrase is an isogram by ignoring non-alphabetic characters.
Go to:
Previous: Write a Python program that accepts a list of numbers. Count the negative numbers and compute the sum of the positive numbers of the said list. Return these values through a list.
Next: Write a Python program to count the number of equal numbers from three given integers.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.