Python: Compute cumulative sum of numbers of a given list
Python Basic - 1: Exercise-92 with Solution
Cumulative Sum of List
Write a Python program to compute the cumulative sum of numbers in a given list.
Note: Cumulative sum = sum of itself + all previous numbers in the said list.
Sample Solution:
Python Code:
# Define a function named nums_cumulative_sum that takes a list of numbers (nums_list) as an argument.
def nums_cumulative_sum(nums_list):
# Use a list comprehension to calculate the cumulative sum for each element in the input list.
# The sum(nums_list[:i+1]) calculates the sum of elements up to the current index i.
# The loop iterates over each index i in the range of the length of the input list.
return [sum(nums_list[:i+1]) for i in range(len(nums_list))]
# Test the function with different lists of numbers and print the results.
# Test case 1
print(nums_cumulative_sum([10, 20, 30, 40, 50, 60, 7]))
# Test case 2
print(nums_cumulative_sum([1, 2, 3, 4, 5]))
# Test case 3
print(nums_cumulative_sum([0, 1, 2, 3, 4, 5]))
Sample Output:
[10, 30, 60, 100, 150, 210, 217] [1, 3, 6, 10, 15] [0, 1, 3, 6, 10, 15]
Explanation:
Here is a breakdown of the above Python code:
- Function definition:
- The code defines a function named "nums_cumulative_sum()" that takes a list of numbers (nums_list) as an argument.
- List comprehension:
- The function uses list comprehension to generate a new list where each element is the cumulative sum of the elements up to the current index.
- Summation Expression:
- The expression sum(nums_list[:i+1]) calculates the sum of elements from index 0 to the current index i.
- Loop Over Indices:
- The loop iterates over each index i in the range of the length of the input list (range(len(nums_list))).
Visual Presentation:
Flowchart:
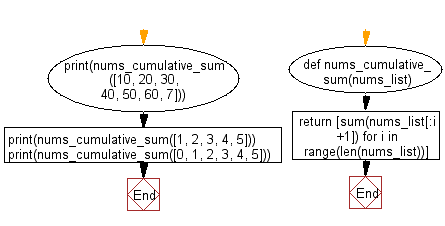
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to count the number of arguments in a given function.
Next: Write a Python program to find the middle character(s) of a given string. If the length of the string is even return the two middle characters. If the length of the string is odd, return the middle character.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/basic/python-basic-1-exercise-92.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics