Python: Check every even index contains an even number and odd index contains odd number of a given list
Even-Odd Index Checker
Write a Python program that checks whether every even index contains an even number and every odd index contains an odd number of a given list.
Sample Solution:
Python Code:
# Define a function named odd_even_position that takes a list of numbers (nums) as an argument.
def odd_even_position(nums):
# Use the all() function along with a generator expression to check if every even index contains an even number
# and every odd index contains an odd number in the given list.
return all(nums[i] % 2 == i % 2 for i in range(len(nums)))
# Test the function with different lists of numbers and print the results.
# Test case 1
nums = [2, 1, 4, 3, 6, 7, 6, 3]
# Print the original list of numbers.
print("Original list of numbers:", nums)
# Print whether every even index contains an even number and every odd index contains an odd number.
print("Check whether every even index contains an even number and every \nodd index contains odd number of a given list:")
print(odd_even_position(nums))
# Test case 2
nums = [2, 1, 4, 3, 6, 7, 6, 4]
# Print the original list of numbers.
print("\nOriginal list of numbers:", nums)
# Print whether every even index contains an even number and every odd index contains an odd number.
print("Check whether every even index contains an even number and every \nodd index contains odd number of a given list:")
print(odd_even_position(nums))
# Test case 3
nums = [4, 1, 2]
# Print the original list of numbers.
print("\nOriginal list of numbers:", nums)
# Print whether every even index contains an even number and every odd index contains an odd number.
print("Check whether every even index contains an even number and every \nodd index contains odd number of a given list:")
print(odd_even_position(nums))
Sample Output:
Original list of numbers: [2, 1, 4, 3, 6, 7, 6, 3] Check whether every even index contains an even number and every odd index contains odd number of a given list: True Original list of numbers: [2, 1, 4, 3, 6, 7, 6, 4] Check whether every even index contains an even number and every odd index contains odd number of a given list: False Original list of numbers: [2, 1, 4, 3, 6, 7, 6, 4] Check whether every even index contains an even number and every odd index contains odd number of a given list: True
Explanation:
Here is a breakdown of the above Python code:
- Function definition:
- The code defines a function named "odd_even_position()" that takes a list of numbers (nums) as an argument.
- all() Function and Generator Expression:
- The function uses the "all()" function along with a generator expression to check if every even index contains an even number and every odd index contains an odd number in the given list.
Visual Presentation:
Flowchart:
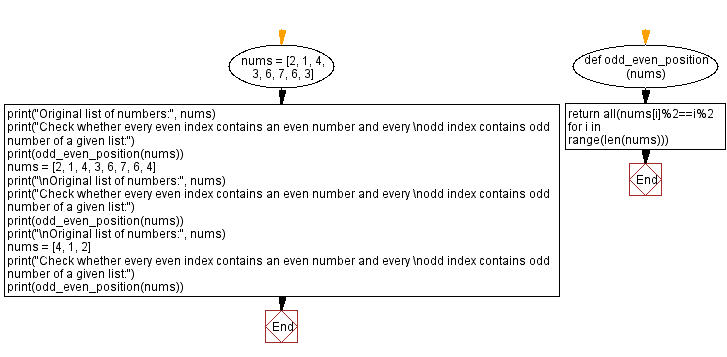
For more Practice: Solve these Related Problems:
- Write a Python program to check if every even-indexed element in a list is even and every odd-indexed element is odd.
- Write a Python program to iterate over a list using enumeration and verify that index parity matches element parity.
- Write a Python program to validate that elements at even positions are even numbers and at odd positions are odd numbers.
- Write a Python program to check a list for the condition that even indices hold even numbers and odd indices hold odd numbers.
Go to:
Previous: Write a Python program to find the largest product of the pair of adjacent elements from a given list of integers.
Next: Write a Python program to check whether a given number is a narcissistic number or not.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.