Python: Check whether a given number is a narcissistic number or not
Python Basic - 1: Exercise-96 with Solution
Write a Python program to check whether a given number is a narcissistic number or not.
If you are a reader of Greek mythology, then you are probably familiar with Narcissus. He was a hunter of exceptional beauty that he died because he was unable to leave a pool after falling in love with his own reflection. That's why I keep myself away from pools these days (kidding).
In mathematics, he has kins by the name of narcissistic numbers — numbers that can't get enough of themselves. In particular, they are numbers that are the sum of their digits when raised to the power of the number of digits.
For example, 371 is a narcissistic number; it has three digits, and if we cube each digits 33 + 73 + 13 the sum is 371. Other 3-digit narcissistic numbers are
153 = 13 + 53 + 33
370 = 33 + 73 + 03
407 = 43 + 03 + 73.
There are also 4-digit narcissistic numbers, some of which are 1634, 8208, 9474 since
1634 = 14+64+34+44
8208 = 84+24+04+84
9474 = 94+44+74+44
It has been proven that there are only 88 narcissistic numbers (in the decimal system) and that the largest of which is
115,132,219,018,763,992,565,095,597,973,971,522,401
has 39 digits.
Ref:: //https://bit.ly/2qNYxo2
Sample Solution:
Python Code:
# Define a function named is_narcissistic_num that takes a number (num) as an argument.
def is_narcissistic_num(num):
# Calculate the sum of each digit raised to the power of the number of digits in the input number.
# Check if the result is equal to the original number.
return num == sum([int(x) ** len(str(num)) for x in str(num)])
# Test the function with different numbers and print the results.
# Test case 1
print(is_narcissistic_num(153))
# Test case 2
print(is_narcissistic_num(370))
# Test case 3
print(is_narcissistic_num(407))
# Test case 4
print(is_narcissistic_num(409))
# Test case 5
print(is_narcissistic_num(1634))
# Test case 6
print(is_narcissistic_num(8208))
# Test case 7
print(is_narcissistic_num(9474))
# Test case 8
print(is_narcissistic_num(9475))
Sample Output:
True True True False True True True False
Explanation:
- Function definition:
- The code defines a function named "is_narcissistic_num()" that takes a number (num) as an argument.
- List comprehension:
- The function uses a list comprehension to calculate the sum of each digit raised to the power of the number of digits in the input number.
- Check for Equality:
- The function checks if the calculated sum is equal to the original number.
- Test cases:
- The function is tested with different numbers using print(is_narcissistic_num(...)).
Visual Presentation:
Flowchart:
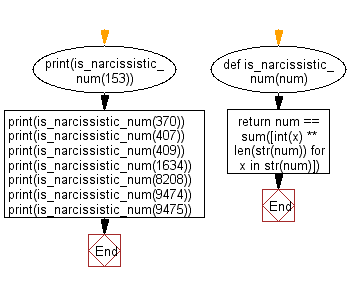
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to check whether every even index contains an even number and every odd index contains odd number of a given list.
Next: Write a Python program to find the highest and lowest number from a given string of space separated integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics