Python Bisect: Find the index position of the last occurrence of a given number in a sorted list using Binary Search
Python Bisect: Exercise-6 with Solution
Write a Python program to find the index position of the last occurrence of a given number in a sorted list using Binary Search (bisect).
Sample Solution:
Python Code:
from bisect import bisect_right
def BinarySearch(a, x):
i = bisect_right(a, x)
if i != len(a)+1 and a[i-1] == x:
return (i-1)
else:
return -1
nums = [1, 2, 3, 4, 8, 8, 10, 12]
x = 8
num_position = BinarySearch(nums, x)
if num_position == -1:
print("not presetn!")
else:
print("Last occurrence of", x, "is present at", num_position)
Sample Output:
Last occurrence of 8 is present at 5
Flowchart:
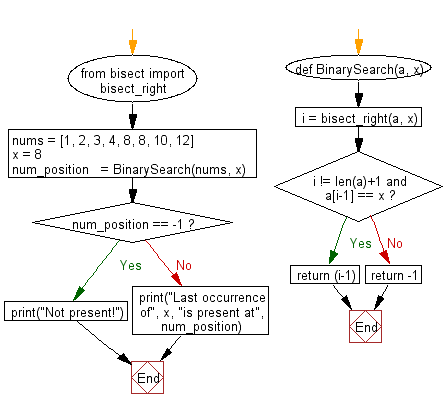
Python Code Editor:
Contribute your code and comments through Disqus.
Next: Write a Python program to find three integers which gives the sum of zero in a given array of integers using Binary Search (bisect).What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/bisect/python-bisect-exercise-6.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics