Python: Check instances and in subclasses a given class
8. Create empty classes Student and Marks, instantiate them, and check instance and subclass relationships
Write a Python program to create two empty classes, Student and Marks. Now create some instances and check whether they are instances of the said classes or not. Also, check whether the said classes are subclasses of the built-in object class or not.
Sample Solution:
Python Code:
class Student:
pass
class Marks:
pass
student1 = Student()
marks1 = Marks()
print(isinstance(student1, Student))
print(isinstance(marks1, Student))
print(isinstance(marks1, Marks))
print(isinstance(student1, Marks))
print("\nCheck whether the said classes are subclasses of the built-in object class or not.")
print(issubclass(Student, object))
print(issubclass(Marks, object))
Sample Output:
True False True False Check whether the said classes are subclasses of the built-in object class or not. True True
Flowchart:
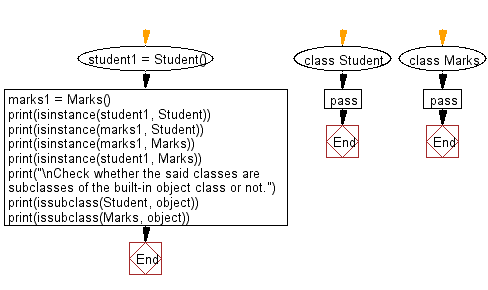
For more Practice: Solve these Related Problems:
- Write a Python program to define two empty classes and create multiple instances, then use isinstance() to check their types.
- Write a Python program to verify that the empty classes are subclasses of the built-in object class.
- Write a Python program to create instances of two empty classes and compare their types using type() and isinstance().
- Write a Python program to implement a function that takes an instance and prints whether it belongs to a specified class and its base classes.
Go to:
Previous: Write a simple Python class named Student and display its type.
Next: Write a Python class named Student with two attributes student_name, marks. Modify the attribute values of the said class and print the original and modified values of the said attributes.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.